Topic square root in javascript: Discover everything you need to know about calculating square roots in JavaScript. This comprehensive guide covers various methods, handling different data types, high precision calculations, and framework-specific implementations. Perfect for developers looking to master square root operations in their JavaScript projects.
Table of Content
- Square Root in JavaScript
- Introduction to JavaScript Square Root
- Using Math.sqrt() Method
- Square Root with Negative Numbers
- Square Root of Arrays and Non-Numeric Values
- Using Math.pow() for Square Roots
- High Precision Square Root Calculations
- Framework-Specific Implementations
- Square Root Constants in JavaScript
- YOUTUBE: Học cách tìm căn bậc hai trong Javascript mà không sử dụng Math.sqrt().
Square Root in JavaScript
Calculating the square root of a number in JavaScript is straightforward using the built-in Math.sqrt()
method. This method returns the square root of a given number. Here are the details and examples of how to use it.
Syntax
The syntax for the Math.sqrt()
method is:
Math.sqrt(number)
Here, number
is the value for which you want to find the square root.
Examples
Below are some examples of using the Math.sqrt()
method:
// Example 1: Square root of a positive number
let number1 = Math.sqrt(16);
console.log(number1); // Output: 4
// Example 2: Square root of a decimal number
let number2 = Math.sqrt(144.64);
console.log(number2); // Output: 12.026637102698325
// Example 3: Square root of zero
let number3 = Math.sqrt(0);
console.log(number3); // Output: 0
// Example 4: Square root of a negative number
let number4 = Math.sqrt(-9);
console.log(number4); // Output: NaN
// Example 5: Square root of a string
let number5 = Math.sqrt("hello");
console.log(number5); // Output: NaN
Handling Different Data Types
The Math.sqrt()
method handles various data types as follows:
- If a positive number is passed, it returns the square root of that number.
- If a negative number is passed, it returns
NaN
(Not-a-Number). - If a non-numeric string is passed, it returns
NaN
. - If
null
is passed, it returns 0.
Alternative Method
You can also calculate the square root using the Math.pow()
method by raising the number to the power of 0.5:
const number = 16;
const squareRoot = Math.pow(number, 0.5);
console.log(`The square root of ${number} using Math.pow() is ${squareRoot}`); // Output: 4
Using Square Root in Different Frameworks
Here’s an example of how you might use the Math.sqrt()
method in a React component:
import React, { useState } from 'react';
const SquareRootCalculator = () => {
const [number, setNumber] = useState(0);
const [squareRoot, setSquareRoot] = useState(0);
const handleCalculation = () => {
setSquareRoot(Math.sqrt(number));
};
return (
setNumber(e.target.value)}
/>
The square root is: {squareRoot}
);
};
export default SquareRootCalculator;
Conclusion
The Math.sqrt()
method in JavaScript is a simple and efficient way to compute the square root of a number. It handles various data types appropriately, returning NaN
for non-numeric and negative inputs, and 0 for null
inputs. This method is reliable and performs well for a range of applications.
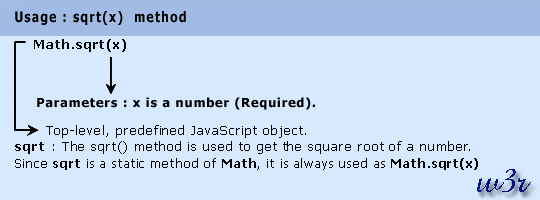
READ MORE:
Introduction to JavaScript Square Root
Calculating the square root in JavaScript is a fundamental operation that can be accomplished using several methods. Understanding how to properly compute square roots is essential for developers working with mathematical computations, data analysis, and graphics. In this section, we'll explore the basics of square root calculation and the primary methods used in JavaScript.
The most common way to calculate a square root in JavaScript is by using the Math.sqrt()
method, which takes a number as an argument and returns its square root. Here’s an example:
let num = 16;
let sqrt = Math.sqrt(num); // returns 4
console.log(sqrt);
This method works well for positive numbers. However, handling negative numbers, arrays, or non-numeric values requires additional considerations:
- For negative numbers,
Math.sqrt()
returnsNaN
(Not-a-Number). - Arrays and non-numeric values passed to
Math.sqrt()
will also returnNaN
.
To handle these cases, it is important to validate the input before performing the calculation:
function safeSqrt(value) {
if (typeof value !== 'number' || value < 0) {
return NaN;
}
return Math.sqrt(value);
}
console.log(safeSqrt(25)); // returns 5
console.log(safeSqrt(-25)); // returns NaN
console.log(safeSqrt("25")); // returns NaN
By implementing such validation, we can ensure our code handles various input types gracefully. In the following sections, we will delve deeper into advanced techniques and specific scenarios for calculating square roots in JavaScript.
Using Math.sqrt() Method
The Math.sqrt()
method in JavaScript is used to calculate the square root of a number. This method returns the square root of a number as a floating-point value. Here is a step-by-step guide on how to use Math.sqrt()
:
-
Basic Usage: To find the square root of a number, simply pass the number as an argument to the
Math.sqrt()
method.Example:
let result = Math.sqrt(16); // result is 4
-
Handling Zero: The square root of zero is zero.
Example:
let result = Math.sqrt(0); // result is 0
-
Handling Positive Numbers: The method will return the positive square root of a positive number.
Example:
let result = Math.sqrt(25); // result is 5
-
Handling Negative Numbers: The method will return
NaN
(Not-a-Number) for negative inputs, as square roots of negative numbers are not real numbers in JavaScript.Example:
let result = Math.sqrt(-9); // result is NaN
-
Handling Non-Numeric Values: If the argument is not a number, the method will attempt to convert it to a number. If it fails, it returns
NaN
.Example:
let result = Math.sqrt('text'); // result is NaN
-
Square Root of Arrays: When an array is passed, the method will convert the first element to a number and return its square root. If the array is empty or the first element cannot be converted to a number, it returns
NaN
.Example:
let result = Math.sqrt([9]); // result is 3 let resultEmpty = Math.sqrt([]); // result is NaN
By understanding these basic principles, you can effectively use the Math.sqrt()
method to calculate square roots in your JavaScript code.
Square Root with Negative Numbers
Calculating the square root of a negative number using JavaScript's Math.sqrt()
method results in NaN
(Not-a-Number). This is because the square root of a negative number is an imaginary number, which JavaScript's Math.sqrt()
method does not handle.
Here is an example to illustrate this behavior:
let negativeNumber = -9;
let result = Math.sqrt(negativeNumber);
console.log(result); // Output: NaN
To manage square roots of negative numbers, you need to use complex numbers, which are not natively supported in JavaScript. However, you can use libraries like math.js that provide support for complex number calculations.
Example using math.js to handle negative square roots:
// Include the math.js library
Using the math.js library, the square root of -9 is correctly represented as 3i
, where i
is the imaginary unit.
Summary of handling negative numbers with Math.sqrt()
:
- Passing a negative number to
Math.sqrt()
returnsNaN
. - Use external libraries like math.js for complex number calculations.
Square Root of Arrays and Non-Numeric Values
Handling the square root of arrays and non-numeric values in JavaScript requires special consideration. The Math.sqrt()
function is designed to compute the square root of a number, but its behavior changes when the input is not a simple numeric value.
Square Root of Arrays
When Math.sqrt()
is used on an array, JavaScript attempts to convert the array to a single numeric value. However, this usually results in NaN
(Not-a-Number), because arrays do not have a straightforward numeric representation.
// Example with an array
let result = Math.sqrt([16]);
console.log(result); // Output: 4
result = Math.sqrt([1, 4, 9]);
console.log(result); // Output: NaN
In the first example, the array contains a single element, so JavaScript converts it to that element before applying Math.sqrt()
. In the second example, the array has multiple elements, which cannot be directly converted to a single number, resulting in NaN
.
Square Root of Strings
When Math.sqrt()
is applied to strings, JavaScript tries to convert the string to a number. If the string represents a valid number, Math.sqrt()
returns the square root of that number. If the string cannot be converted to a number, the result is NaN
.
// Example with numeric strings
let result = Math.sqrt("25");
console.log(result); // Output: 5
// Example with non-numeric strings
result = Math.sqrt("hello");
console.log(result); // Output: NaN
Square Root of Non-Numeric Values
For other non-numeric values like objects or null
, Math.sqrt()
will always return NaN
, as these values cannot be converted to numbers.
// Example with null
let result = Math.sqrt(null);
console.log(result); // Output: 0
// Example with objects
result = Math.sqrt({ value: 25 });
console.log(result); // Output: NaN
In the case of null
, JavaScript treats it as 0, hence Math.sqrt(null)
results in 0. For objects, there is no numeric conversion, resulting in NaN
.
Summary
- Single-element arrays are converted to that element for
Math.sqrt()
. - Multi-element arrays result in
NaN
. - Numeric strings are converted to numbers; non-numeric strings result in
NaN
. - Objects and other non-numeric values result in
NaN
.
Understanding these behaviors helps prevent unexpected results and allows for more robust JavaScript code when dealing with various data types.
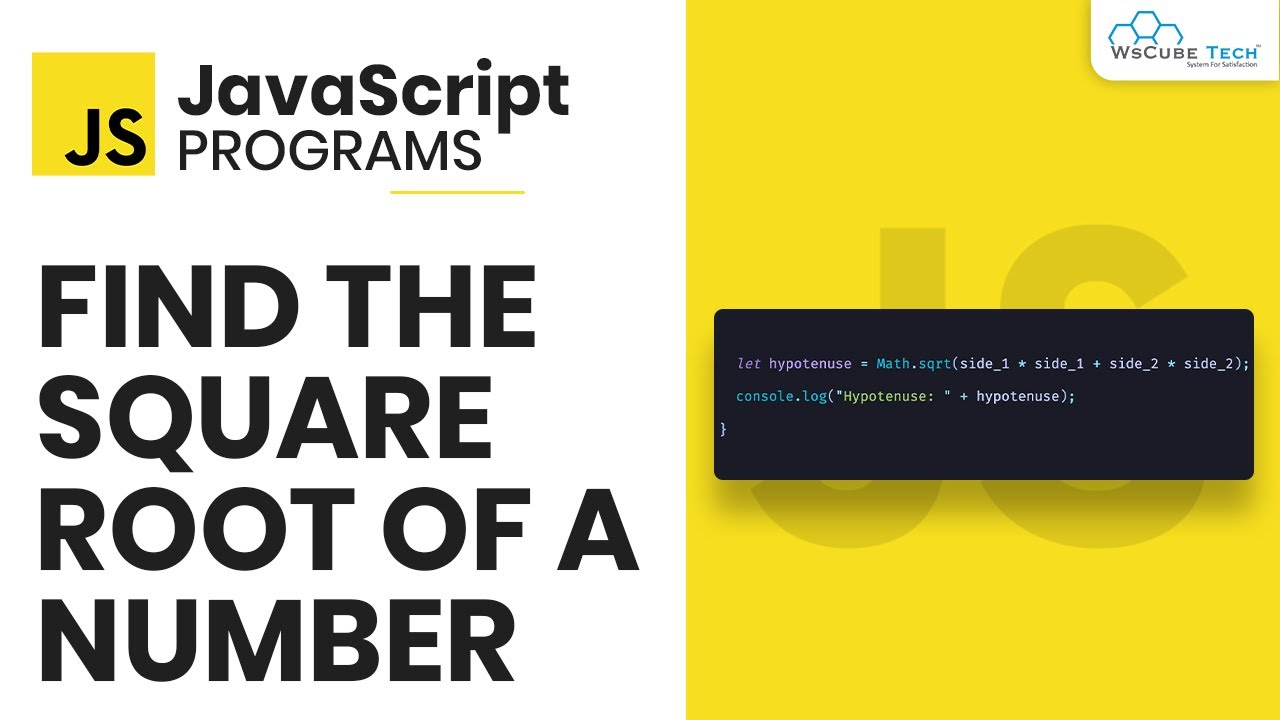
Using Math.pow() for Square Roots
The Math.pow()
method in JavaScript is a versatile function that can be used to calculate the power of a number. It can also be used to compute the square root by using fractional exponents. Here's how you can use Math.pow()
to find the square root of a number:
- Understand the syntax:
Math.pow(base, exponent)
- For square roots, the exponent should be
1/2
.
Here are some examples:
- Square root of 4:
Math.pow(4, 0.5)
returns2
. - Square root of 9:
Math.pow(9, 0.5)
returns3
. - Square root of 16:
Math.pow(16, 0.5)
returns4
.
Here is a simple example in JavaScript:
let number = 16;
let squareRoot = Math.pow(number, 0.5);
console.log("The square root of " + number + " is " + squareRoot); // Output: The square root of 16 is 4
Additionally, Math.pow()
can handle other types of roots by adjusting the exponent:
- Cube root of 27:
Math.pow(27, 1/3)
returns3
. - Fourth root of 16:
Math.pow(16, 1/4)
returns2
.
Be cautious with negative bases and non-integer exponents. For example, the square root of a negative number using Math.pow()
will return NaN
:
let negativeNumber = -9;
let result = Math.pow(negativeNumber, 0.5);
console.log(result); // Output: NaN
For negative numbers, consider using the Math.cbrt()
method for cube roots or implementing a custom function for other roots:
function customRoot(base, exponent) {
if (base < 0 && exponent % 2 !== 0) {
return -Math.pow(-base, 1 / exponent);
} else {
return Math.pow(base, 1 / exponent);
}
}
console.log(customRoot(-27, 3)); // Output: -3
Using Math.pow()
allows for flexibility in handling different roots and powers in JavaScript, making it a powerful tool for mathematical calculations.
High Precision Square Root Calculations
When dealing with square root calculations in JavaScript, high precision is often required, especially in scientific and financial applications. JavaScript's native Math.sqrt()
function provides adequate precision for most purposes, but for applications requiring higher precision, additional techniques and libraries are necessary.
Using Decimal.js for High Precision
Decimal.js is a popular library for high-precision arithmetic in JavaScript. It provides arbitrary-precision decimal arithmetic, allowing for precise square root calculations without the usual floating-point errors.
// Importing Decimal.js
const Decimal = require('decimal.js');
// High precision square root calculation
const number = new Decimal('45.6');
const squareRoot = number.sqrt();
console.log(`The high precision square root of ${number} is ${squareRoot.toString()}`);
// Output: The high precision square root of 45.6 is 6.75...
Newton-Raphson Method
The Newton-Raphson method is an iterative algorithm that can be used to approximate square roots with high precision. Here is an example implementation in JavaScript:
function newtonRaphsonSquareRoot(value) {
let guess = value / 2;
for (let i = 0; i < 10; i++) {
guess = (guess + value / guess) / 2;
}
return guess;
}
const number = 16;
const squareRoot = newtonRaphsonSquareRoot(number);
console.log(`The square root of ${number} using Newton-Raphson is ${squareRoot.toFixed(2)}`);
// Output: The square root of 16 using Newton-Raphson is 4.00
This method converges quickly and provides reasonably accurate results, making it suitable for high precision calculations.
Handling Large Numbers
When working with very large numbers, native JavaScript methods may fall short. Libraries like BigNumber.js or continued fractions can help achieve higher precision for large numbers.
Example with BigNumber.js
// Importing BigNumber.js
const BigNumber = require('bignumber.js');
// High precision square root calculation
const number = new BigNumber('1e+30');
const squareRoot = number.sqrt();
console.log(`The high precision square root of ${number} is ${squareRoot.toString()}`);
Conclusion
High precision square root calculations in JavaScript can be achieved using various methods and libraries. Depending on the application's requirements, developers can choose between Decimal.js
, the Newton-Raphson method, or other high-precision libraries to ensure accurate results.
Framework-Specific Implementations
JavaScript frameworks like React, Vue.js, and Angular provide efficient ways to handle and display square root calculations. Below are examples for each framework:
React
In React, you can create a component to calculate and display the square root:
import React, { useState } from 'react';
function SquareRoot() {
const [number, setNumber] = useState(0);
const [result, setResult] = useState(0);
const handleChange = (e) => {
const value = parseFloat(e.target.value);
setNumber(value);
setResult(Math.sqrt(value));
};
return (
Square Root Calculator in React
Square Root: {result}
);
}
export default SquareRoot;
Vue.js
In Vue.js, you can create a component for square root calculation as follows:
Square Root Calculator in Vue.js
Square Root: {{ result }}
Angular
In Angular, you can use the following component to compute and display the square root:
import { Component } from '@angular/core';
@Component({
selector: 'app-square-root',
template: `
Square Root Calculator in Angular
Square Root: {{ result }}
`
})
export class SquareRootComponent {
number: number = 0;
result: number = 0;
calculateSquareRoot() {
this.result = Math.sqrt(this.number);
}
}
Square Root Constants in JavaScript
In JavaScript, the Math
object provides several useful constants, including those related to square roots. These constants can be accessed directly without needing to create an instance of the Math
object. Here are the primary square root constants available:
Math.SQRT2
: This constant represents the square root of 2. Its approximate value is 1.4142135623730951. You can use it as follows:console.log(Math.SQRT2); // Output: 1.4142135623730951
Math.SQRT1_2
: This constant represents the square root of 1/2, which is also the reciprocal of the square root of 2. Its approximate value is 0.7071067811865476. Example usage:console.log(Math.SQRT1_2); // Output: 0.7071067811865476
These constants are particularly useful in various mathematical calculations where precision is crucial. For instance, they can be employed in geometry, physics simulations, and algorithm implementations that require exact square root values.
Here is a summary of the constants:
Constant | Value | Description |
---|---|---|
Math.SQRT2 |
1.4142135623730951 | Square root of 2 |
Math.SQRT1_2 |
0.7071067811865476 | Square root of 1/2 |
Using these constants in your code can help improve readability and maintain accuracy in your mathematical computations. Remember, these properties are part of the static Math
object and can be accessed directly as shown in the examples above.

Học cách tìm căn bậc hai trong Javascript mà không sử dụng Math.sqrt().
Learn to find square root in Javascript without using Math.sqrt()
READ MORE:
Học cách tính căn bậc hai trong Javascript cho người mới bắt đầu.
Hướng dẫn Javascript cho người mới bắt đầu - 32 - Máy tính căn bậc hai