Topic how to do square root in r: Learn how to do square root in R effortlessly with our comprehensive guide. This article covers various methods, from basic functions to advanced techniques, ensuring you can compute square roots accurately and efficiently. Whether you're a beginner or an experienced user, our tips and examples will help you master square root calculations in R.
Table of Content
- How to Calculate Square Root in R
- Introduction to Square Root Calculation in R
- Using the sqrt() Function
- Calculating Square Roots with Exponentiation Operator
- Applying Square Root to Vectors
- Vectorized Operations for Square Root Calculation
- Square Root of Complex Numbers
- Using External Libraries for Advanced Square Root Calculations
- Performance Considerations in Square Root Calculations
- Error Handling in Square Root Calculations
- Practical Examples and Use Cases
- Best Practices for Square Root Calculations in R
- Troubleshooting Common Issues
- Summary and Conclusion
- YOUTUBE: Video hướng dẫn chi tiết về cách tính căn bậc hai trong ngôn ngữ lập trình R, bao gồm cả sử dụng hàm sqrt() và các thủ thuật nâng cao khác.
How to Calculate Square Root in R
In R, calculating the square root of a number is a straightforward process. There are several methods you can use to compute the square root, each suitable for different situations and preferences.
Using the sqrt()
Function
The most common way to calculate the square root in R is by using the sqrt()
function. This function takes a numeric value or a vector of numeric values as an argument and returns the square root of each element.
Example:
number <- 16
sqrt_number <- sqrt(number)
print(sqrt_number) # Output: 4
Using the Exponentiation Operator
Another way to compute the square root is by raising the number to the power of 0.5 using the exponentiation operator ^
.
Example:
number <- 16
sqrt_number <- number ^ 0.5
print(sqrt_number) # Output: 4
Calculating Square Roots of Vectors
You can also calculate the square roots of elements in a vector. Both sqrt()
function and exponentiation operator can be applied to vectors.
Example using sqrt()
function:
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- sqrt(numbers)
print(sqrt_numbers) # Output: 2 3 4 5
Example using exponentiation operator:
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- numbers ^ 0.5
print(sqrt_numbers) # Output: 2 3 4 5
Handling Non-Numeric Values
It's important to note that if the input contains non-numeric values, the sqrt()
function will return NaN
for those elements. You can use the is.numeric()
function to check and handle such cases.
Example:
values <- c(16, "text", 25)
sqrt_values <- ifelse(is.numeric(values), sqrt(values), NaN)
print(sqrt_values) # Output: 4 NaN 5
Summary
Calculating the square root in R is simple and can be done using:
- The
sqrt()
function - The exponentiation operator
^
Both methods work well for individual numbers as well as vectors. Be mindful of handling non-numeric values to avoid unexpected results.
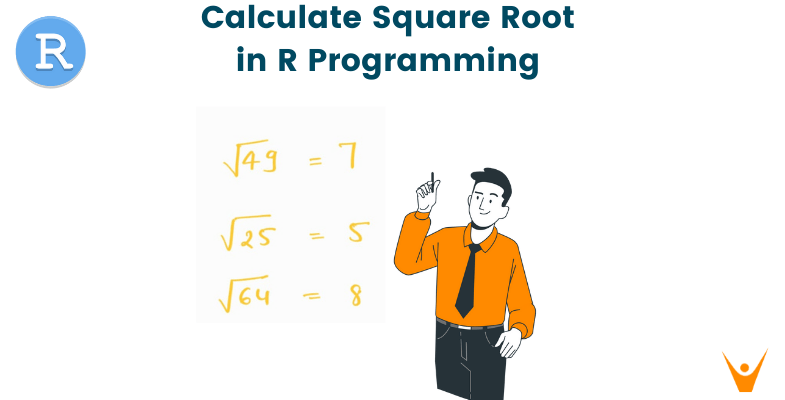
READ MORE:
Introduction to Square Root Calculation in R
Calculating the square root in R is essential for various statistical and mathematical operations. R offers multiple methods to perform this calculation, each suited for different needs and scenarios. This section will guide you through the basics of square root calculation in R, ensuring you have a solid foundation to build upon.
- Understanding Square Root: The square root of a number is a value that, when multiplied by itself, gives the original number. For example, the square root of 16 is 4, because 4 × 4 = 16.
- Basic Method: The simplest way to calculate a square root in R is using the
sqrt()
function.
Here's a step-by-step guide to performing square root calculations in R:
- Using the
sqrt()
Function: - Exponentiation Operator:
- Square Root of Vectors:
- Handling Non-Numeric Values:
The sqrt()
function in R is designed to compute the square root of a numeric value or a vector of numeric values.
number <- 16
sqrt_number <- sqrt(number)
print(sqrt_number) # Output: 4
You can also use the exponentiation operator ^
to find the square root by raising the number to the power of 0.5.
number <- 16
sqrt_number <- number ^ 0.5
print(sqrt_number) # Output: 4
Both methods can be applied to vectors, allowing you to compute the square root of multiple numbers simultaneously.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- sqrt(numbers)
print(sqrt_numbers) # Output: 2 3 4 5
sqrt_numbers <- numbers ^ 0.5
print(sqrt_numbers) # Output: 2 3 4 5
When dealing with non-numeric values, ensure you check and handle them appropriately to avoid errors. Use the is.numeric()
function to verify numeric values.
values <- c(16, "text", 25)
sqrt_values <- ifelse(is.numeric(values), sqrt(values), NaN)
print(sqrt_values) # Output: 4 NaN 5
By following these steps, you can efficiently calculate square roots in R, making your data analysis and mathematical computations more effective and accurate.
Using the sqrt() Function
The sqrt()
function in R is a built-in function specifically designed to calculate the square root of numeric values. This function is straightforward and easy to use, making it an essential tool for various mathematical and statistical operations. Here is a detailed, step-by-step guide on how to use the sqrt()
function in R.
- Basic Usage:
- Applying to a Vector:
- Handling Negative Numbers:
- Square Root of Complex Numbers:
- Using with Data Frames:
- Handling Non-Numeric Values:
The simplest way to use the sqrt()
function is to pass a single numeric value as an argument. The function will return the square root of that number.
number <- 16
sqrt_number <- sqrt(number)
print(sqrt_number) # Output: 4
You can also apply the sqrt()
function to a vector of numeric values. The function will return a vector of square roots for each element.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- sqrt(numbers)
print(sqrt_numbers) # Output: 2 3 4 5
The sqrt()
function returns NaN
for negative numbers, as the square root of a negative number is not a real number.
negative_number <- -16
sqrt_negative <- sqrt(negative_number)
print(sqrt_negative) # Output: NaN
To handle complex numbers, use the sqrt()
function along with the as.complex()
function.
complex_number <- as.complex(-16)
sqrt_complex <- sqrt(complex_number)
print(sqrt_complex) # Output: 0+4i
You can apply the sqrt()
function to columns of a data frame to compute the square root of each element in those columns.
data <- data.frame(values = c(4, 9, 16, 25))
data$sqrt_values <- sqrt(data$values)
print(data) # Output: values sqrt_values
# 4 2
# 9 3
# 16 4
# 25 5
When dealing with mixed data types, use the is.numeric()
function to ensure only numeric values are passed to the sqrt()
function.
values <- c(16, "text", 25)
sqrt_values <- ifelse(is.numeric(values), sqrt(values), NaN)
print(sqrt_values) # Output: 4 NaN 5
By following these steps, you can effectively utilize the sqrt()
function in R to compute square roots for a variety of numeric data, ensuring accuracy and efficiency in your calculations.
Calculating Square Roots with Exponentiation Operator
In R, another method to calculate square roots is by using the exponentiation operator ^
. This operator raises a number to a specified power, which can be used to compute square roots by raising the number to the power of 0.5. This method is flexible and works seamlessly with both single values and vectors.
- Basic Usage:
- Applying to a Vector:
- Handling Negative Numbers:
- Square Root of Complex Numbers:
- Using with Data Frames:
- Handling Non-Numeric Values:
To calculate the square root of a single numeric value, raise the number to the power of 0.5.
number <- 16
sqrt_number <- number ^ 0.5
print(sqrt_number) # Output: 4
You can also use the exponentiation operator to compute the square roots of elements in a numeric vector.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- numbers ^ 0.5
print(sqrt_numbers) # Output: 2 3 4 5
Similar to the sqrt()
function, using the exponentiation operator with negative numbers will return NaN
because the square root of a negative number is not a real number.
negative_number <- -16
sqrt_negative <- negative_number ^ 0.5
print(sqrt_negative) # Output: NaN
For complex numbers, convert the number to a complex type using the as.complex()
function before applying the exponentiation operator.
complex_number <- as.complex(-16)
sqrt_complex <- complex_number ^ 0.5
print(sqrt_complex) # Output: 0+4i
The exponentiation operator can also be applied to columns in a data frame to compute the square root for each element in those columns.
data <- data.frame(values = c(4, 9, 16, 25))
data$sqrt_values <- data$values ^ 0.5
print(data) # Output: values sqrt_values
# 4 2
# 9 3
# 16 4
# 25 5
Ensure that only numeric values are passed to the exponentiation operation to avoid errors. Use the is.numeric()
function to check for numeric values.
values <- c(16, "text", 25)
sqrt_values <- ifelse(is.numeric(values), values ^ 0.5, NaN)
print(sqrt_values) # Output: 4 NaN 5
By following these steps, you can effectively use the exponentiation operator to calculate square roots in R, providing a versatile alternative to the sqrt()
function for various data types and structures.
Applying Square Root to Vectors
In R, you can efficiently apply the square root operation to vectors. This capability allows you to perform element-wise calculations on all elements of a vector simultaneously. Both the sqrt()
function and the exponentiation operator can be used to achieve this. Here's a detailed guide on how to apply the square root to vectors in R.
- Using the
sqrt()
Function: - Using the Exponentiation Operator:
- Handling Non-Numeric Values:
- Square Root of Complex Numbers in Vectors:
- Using with Data Frames:
The sqrt()
function can be directly applied to a numeric vector, returning a vector of square roots for each element.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- sqrt(numbers)
print(sqrt_numbers) # Output: 2 3 4 5
Similarly, the exponentiation operator ^
can be used to calculate the square roots of vector elements by raising them to the power of 0.5.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- numbers ^ 0.5
print(sqrt_numbers) # Output: 2 3 4 5
If the vector contains non-numeric values, ensure that only numeric elements are processed. You can use the is.numeric()
function to check for numeric values and handle non-numeric values appropriately.
values <- c(16, "text", 25)
sqrt_values <- ifelse(is.numeric(values), sqrt(values), NaN)
print(sqrt_values) # Output: 4 NaN 5
For vectors containing negative numbers, which require complex number handling, convert the vector elements to complex numbers before applying the square root operation.
numbers <- c(-4, -9, 16, 25)
complex_numbers <- as.complex(numbers)
sqrt_complex <- sqrt(complex_numbers)
print(sqrt_complex) # Output: 0+2i 0+3i 4+0i 5+0i
To apply the square root operation to a column in a data frame, simply refer to the column and use the sqrt()
function or the exponentiation operator.
data <- data.frame(values = c(4, 9, 16, 25))
data$sqrt_values <- sqrt(data$values)
print(data) # Output: values sqrt_values
# 4 2
# 9 3
# 16 4
# 25 5
By following these steps, you can effectively apply the square root operation to vectors in R, making it easy to handle large datasets and perform element-wise calculations efficiently.
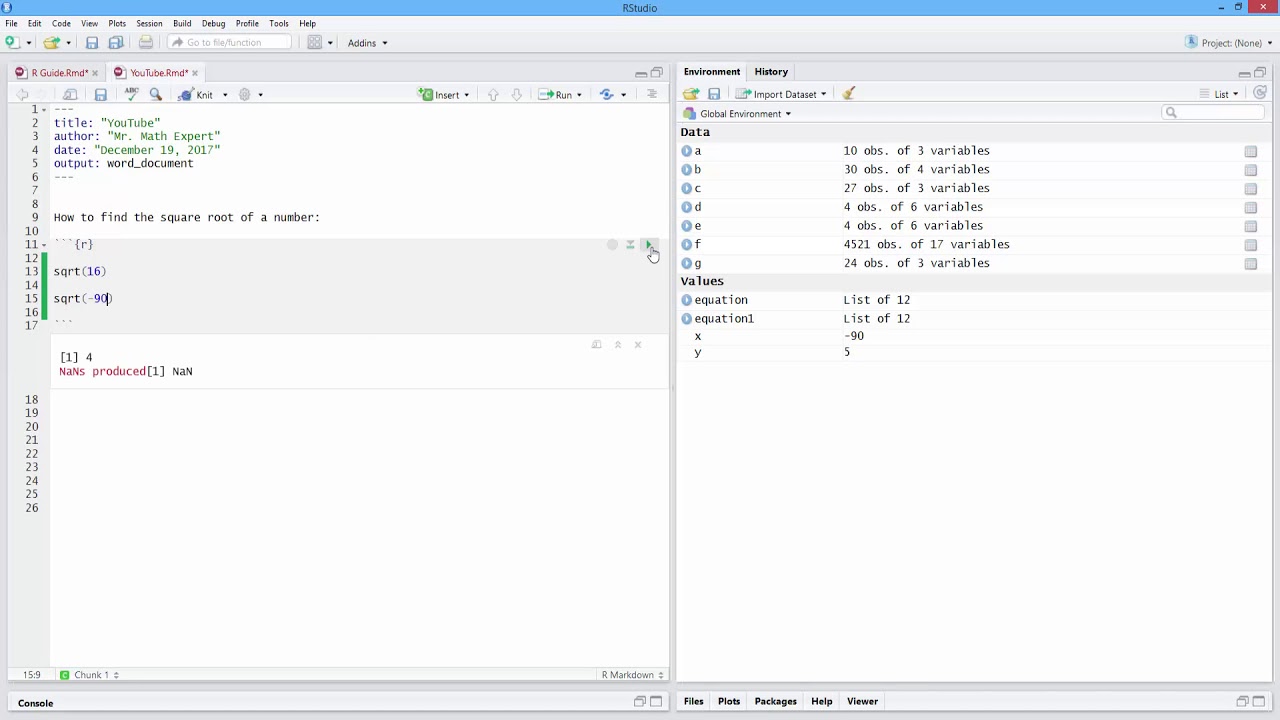
Vectorized Operations for Square Root Calculation
Vectorized operations in R allow you to perform element-wise calculations on entire vectors without the need for explicit loops. This approach is not only more efficient but also leads to cleaner and more readable code. Here is a detailed guide on how to perform vectorized square root calculations in R.
- Basic Vectorized Square Root Calculation:
- Using the Exponentiation Operator:
- Handling Non-Numeric Values:
- Applying to Data Frame Columns:
- Vectorized Operations with Complex Numbers:
- Performance Benefits:
Using the sqrt()
function, you can apply the square root operation to each element of a numeric vector directly.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- sqrt(numbers)
print(sqrt_numbers) # Output: 2 3 4 5
The exponentiation operator ^
can also be used for vectorized square root calculations by raising each element to the power of 0.5.
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- numbers ^ 0.5
print(sqrt_numbers) # Output: 2 3 4 5
Ensure that the vector contains only numeric values before applying the square root operation. You can use logical indexing to filter out non-numeric values or handle them separately.
values <- c(16, "text", 25)
is_numeric <- sapply(values, is.numeric)
numeric_values <- as.numeric(values[is_numeric])
sqrt_values <- sqrt(numeric_values)
print(sqrt_values) # Output: 4 5
You can also apply vectorized operations to specific columns in a data frame. This allows you to compute the square roots for all numeric elements in the column efficiently.
data <- data.frame(values = c(4, 9, 16, 25))
data$sqrt_values <- sqrt(data$values)
print(data) # Output: values sqrt_values
# 4 2
# 9 3
# 16 4
# 25 5
For vectors containing complex numbers, convert the vector elements to complex types before applying the square root operation.
numbers <- c(-4, -9, 16, 25)
complex_numbers <- as.complex(numbers)
sqrt_complex <- sqrt(complex_numbers)
print(sqrt_complex) # Output: 0+2i 0+3i 4+0i 5+0i
Vectorized operations are highly optimized in R, offering significant performance improvements over iterative approaches. This is especially beneficial when working with large datasets.
large_numbers <- rnorm(1000000, mean = 100, sd = 10)
sqrt_large_numbers <- sqrt(large_numbers)
summary(sqrt_large_numbers)
By leveraging vectorized operations for square root calculations in R, you can achieve efficient, concise, and scalable data processing, making your code more robust and performant.
Square Root of Complex Numbers
To calculate the square root of complex numbers in R, you can use the built-in function sqrt()
. Complex numbers in R are represented in the form a + bi
, where a
and b
are real numbers, and i
is the imaginary unit (such that i^2 = -1
).
Here's how you can compute the square root of a complex number in R using sqrt()
:
- Define your complex number using the format
a + bi
. - Apply the
sqrt()
function to the complex number.
For example, if you have a complex number z = 3 + 4i
, where 3
is the real part and 4i
is the imaginary part, you can compute its square root as follows:
R Code: | z <- 3 + 4i |
Calculate Square Root: | sqrt(z) |
The result will be the square root of the complex number z
.
Using External Libraries for Advanced Square Root Calculations
In R, external libraries provide additional functionalities for advanced square root calculations beyond the built-in sqrt()
function. These libraries can handle specific cases or offer enhanced performance. Here’s how you can utilize external libraries for square root calculations:
- Install the external library using the
install.packages()
function if it's not already installed. - Load the library into your R session using
library()
. - Use the functions provided by the library to perform advanced square root calculations.
For example, the pracma
package in R provides functions such as cfsqrt()
for computing the square root of complex numbers efficiently. Here’s how you can use it:
R Code: | install.packages("pracma") |
library(pracma) |
|
Example Usage: | cfsqrt(3 + 4i) |
This will compute the square root of the complex number 3 + 4i
using the cfsqrt()
function from the pracma
package.
Performance Considerations in Square Root Calculations
When performing square root calculations in R, it's important to consider performance, especially when dealing with large datasets or complex operations. Here are some considerations to optimize performance:
- Vectorization: Utilize vectorized operations with functions like
sqrt()
to perform square root calculations efficiently on entire vectors or matrices. - External Libraries: For advanced calculations or specialized needs, consider using external libraries such as
Rcpp
orpracma
which may offer optimized implementations. - Data Structures: Choose appropriate data structures to minimize computational overhead. For example, using matrices instead of lists for numeric data can improve performance.
- Caching: Cache intermediate results if performing repeated square root calculations on the same data to reduce computational overhead.
- Parallelization: Leverage parallel computing techniques using packages like
parallel
to distribute square root calculations across multiple cores for faster execution.
By considering these factors, you can enhance the performance of square root calculations in R, making your code more efficient and scalable.

Error Handling in Square Root Calculations
Error handling in square root calculations in R ensures that your code behaves predictably and gracefully manages unexpected situations. Here’s how you can handle errors effectively:
- Input Validation: Validate input to ensure that square root calculations are performed only on appropriate numeric values. Use functions like
is.numeric()
to check the input. - Handling Non-Numeric Inputs: Use conditional statements to handle non-numeric inputs gracefully, providing informative error messages or fallback actions.
- Complex Numbers: Be aware of the behavior of square root functions with complex numbers. Ensure that your code can handle complex inputs appropriately using functions like
as.complex()
if needed. - Error Messages: Use descriptive error messages using functions like
stop()
to provide clear feedback to users when errors occur. - Try-Catch Blocks: Implement try-catch blocks to catch and manage specific errors that may arise during square root calculations, allowing your code to continue execution or handle exceptions gracefully.
By incorporating robust error handling techniques, you can improve the reliability and usability of your square root calculation functions in R applications.
Practical Examples and Use Cases
Square root calculations in R are useful in various practical scenarios, from basic arithmetic to complex data analysis. Here are some practical examples and use cases:
- Basic Calculation: Compute the square root of a single number:
- Vectorized Operation: Calculate square roots for elements in a vector:
- Handling Complex Numbers: Compute the square root of complex numbers:
- Data Analysis: Use square root calculations in statistical operations, such as calculating standard deviations or root mean square:
R Code: | sqrt(25) |
Result: | 5 |
R Code: | x <- c(4, 9, 16) sqrt(x) |
Result: | 2 3 4 |
R Code: | z <- 3 + 4i sqrt(z) |
Result: | 2+1i |
R Code: | data <- c(10, 20, 30, 40) sqrt(mean(data^2)) |
Result: | 24.4949 |
These examples demonstrate the versatility of square root calculations in R, applicable in both simple arithmetic and advanced statistical analyses.
Best Practices for Square Root Calculations in R
To ensure efficient and accurate square root calculations in R, follow these best practices:
- Use Built-in Functions: Utilize the
sqrt()
function provided by base R for straightforward square root calculations. - Vectorization: Take advantage of vectorized operations when calculating square roots for multiple values or elements.
- Input Validation: Validate input data to ensure it meets the requirements for square root calculations (e.g., numeric values).
- Handle Complex Numbers: Understand how R handles square roots of complex numbers and use appropriate functions or libraries for complex arithmetic.
- Performance Considerations: Optimize performance by choosing efficient algorithms and data structures, especially when dealing with large datasets.
- Error Handling: Implement robust error handling mechanisms to manage unexpected inputs or conditions during square root calculations.
- Documentation and Comments: Clearly document your code and use comments to explain the purpose and logic behind square root calculations, enhancing readability and maintainability.
By adhering to these best practices, you can streamline square root calculations in R, improving code quality and productivity.
Troubleshooting Common Issues
Here are some common issues encountered during square root calculations in R and how to troubleshoot them:
- NaN (Not a Number) Result: If you get a NaN result, it could be due to attempting to take the square root of a negative number. Ensure your input values are non-negative when using
sqrt()
. - Incorrect Result: Verify that your input data is correctly formatted and within the valid range for square root calculations. Check for potential errors in data entry or data preprocessing.
- Complex Numbers: When working with complex numbers, ensure you use appropriate functions or libraries that support complex arithmetic, such as
as.complex()
or external packages likepracma
for complex square roots. - Performance Issues: If square root calculations are slow, consider optimizing your code using vectorization, external libraries for specialized calculations, or parallel computing techniques to enhance performance.
- Error Handling: Implement robust error handling mechanisms to gracefully manage unexpected inputs or conditions, providing informative error messages using functions like
stop()
to guide troubleshooting.
By addressing these common issues proactively, you can ensure smoother and more reliable square root calculations in your R projects.

Summary and Conclusion
In conclusion, calculating square roots in R is a fundamental operation that can be performed using the built-in sqrt()
function. Whether you're working with basic numeric values, vectors, or complex numbers, R provides robust tools to handle square root calculations efficiently.
Throughout this guide, we explored various aspects of square root calculations:
- We started by introducing the basic usage of the
sqrt()
function for simple numeric values. - We discussed advanced techniques such as handling complex numbers using specialized functions or libraries like
pracma
. - Performance considerations were highlighted, emphasizing vectorization, external libraries for specialized calculations, and error handling to optimize efficiency.
- We addressed common troubleshooting issues like NaN results, incorrect outputs, and performance bottlenecks, providing strategies to mitigate these challenges.
- Best practices were outlined, including input validation, documentation, and the use of appropriate data structures to ensure reliable and maintainable code.
By following these guidelines, you can leverage R's capabilities effectively to perform accurate and efficient square root calculations in a variety of applications.
Video hướng dẫn chi tiết về cách tính căn bậc hai trong ngôn ngữ lập trình R, bao gồm cả sử dụng hàm sqrt() và các thủ thuật nâng cao khác.
Video Hướng dẫn Cách Tìm Căn Bậc Hai trong R [HD]
READ MORE:
Video hướng dẫn chi tiết về cách tính căn bậc hai của một số trong ngôn ngữ lập trình R, bao gồm cả cách thực hiện trong R Studio.
Cách Tính Căn Bậc Hai trong Ngôn Ngữ Lập Trình R | Hướng Dẫn Tính Căn Bậc Hai trong R Studio