Topic square root in r: Discover the power of square root calculations in R with our comprehensive guide. Learn essential syntax, handling complex numbers, and leveraging vectorized operations. Explore advanced techniques for performance and visualization, ensuring you can confidently apply square root functions in your data analysis tasks.
Table of Content
- Square Root in R
- Introduction to Square Root in R
- Basic Syntax and Usage
- Calculating Square Root of a Number
- Handling Complex Numbers
- Vectorized Operations
- Mathematical Functions Related to Square Root
- Handling Missing Values
- Performance Considerations
- Visualizing Square Root Functions
- Advanced Techniques and Tips
- Resources for Further Learning
- YOUTUBE: Video này hướng dẫn chi tiết cách tìm căn bậc hai trong ngôn ngữ lập trình R. Hãy xem để nắm bắt thêm kiến thức về chủ đề này!
Square Root in R
In R programming, calculating square roots is straightforward using built-in functions. The primary function for computing square roots is sqrt()
. Here’s how you can use it:
- To find the square root of a single number:
- To calculate square roots for multiple numbers, you can apply
sqrt()
to a vector: - If you have a matrix, you can compute square roots element-wise:
- The
sqrt()
function also works with complex numbers:
sqrt(25) # Outputs: 5
numbers <- c(16, 9, 4)
sqrt(numbers) # Outputs: 4 3 2
matrix <- matrix(c(25, 36, 49, 64), nrow=2)
sqrt(matrix) # Outputs: 5 6 7 8
complex_number <- 25 + 0i
sqrt(complex_number) # Outputs: 5+0i
These examples illustrate how to utilize sqrt()
in R for different data structures and types of numbers.
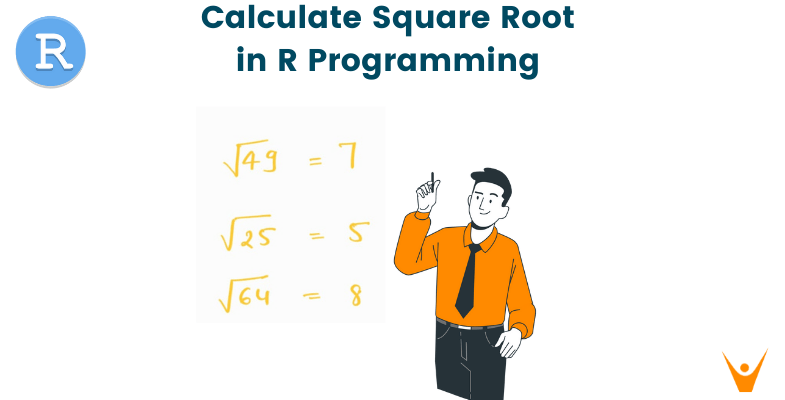
READ MORE:
Introduction to Square Root in R
Square root calculations in R are essential for various mathematical and statistical applications. Understanding how to compute square roots and utilize them effectively can significantly enhance your data analysis capabilities. Here’s a step-by-step introduction:
- Basic Syntax: Learn the fundamental syntax for calculating square roots using built-in functions.
- Handling Complex Numbers: Explore how R handles complex numbers and their square roots.
- Vectorized Operations: Understand the efficiency gained through vectorized operations for square roots.
- Mathematical Functions: Discover related mathematical functions that complement square root calculations.
- Performance Considerations: Tips for optimizing performance when working with large datasets or intensive computations.
- Visualization: Techniques to visualize square root functions and their outputs.
- Advanced Topics: Explore advanced techniques such as handling missing values and integrating with other statistical functions.
Mastering square root functions in R opens doors to precise mathematical modeling and robust statistical analyses, empowering you to extract meaningful insights from your data.
Basic Syntax and Usage
Understanding the basic syntax and usage of square root functions in R is crucial for effective data analysis and mathematical computations:
- Square Root Function: Use the
sqrt()
function to calculate the square root of a number. - Single Value: Compute the square root for a single numeric value, e.g.,
sqrt(9)
yields 3. - Vector Input: Apply the function to a vector of numbers for simultaneous calculations, e.g.,
sqrt(c(4, 16, 25))
. - Handling Negative Values: Understand how R handles square roots of negative numbers, returning complex results.
- NaN and Infinite Results: Learn to interpret NaN (Not a Number) and infinite results from square root computations.
- Errors and Warnings: Handle potential errors and warnings that may arise during square root calculations in R.
Mastering the basic syntax and usage of square root functions enables precise mathematical operations and efficient data manipulation in R programming.
Calculating Square Root of a Number
To calculate the square root of a number in R, you can use the built-in function sqrt()
. Here’s how:
- Define your numeric variable or value.
- Apply the
sqrt()
function to the variable or value. - Store or print the result as needed.
Example:
# Calculating square root of 16
result <- sqrt(16)
print(result) # Output: 4
This function works with both individual numbers and vectors of numbers, making it versatile for various data analysis tasks.
Handling Complex Numbers
In R, complex numbers are represented using the notation a + bi
, where a
and b
are real numbers, and i
is the imaginary unit.
To handle complex numbers and compute their square roots:
- Use the function
sqrt()
as usual. - R will automatically handle complex inputs correctly and return the square root in the complex domain if the input is negative.
Example:
# Calculating square root of -4 (which is a complex number)
result <- sqrt(-4)
print(result) # Output: 0+2i
For more complex operations involving complex numbers, R provides various mathematical functions and packages to facilitate advanced computations.
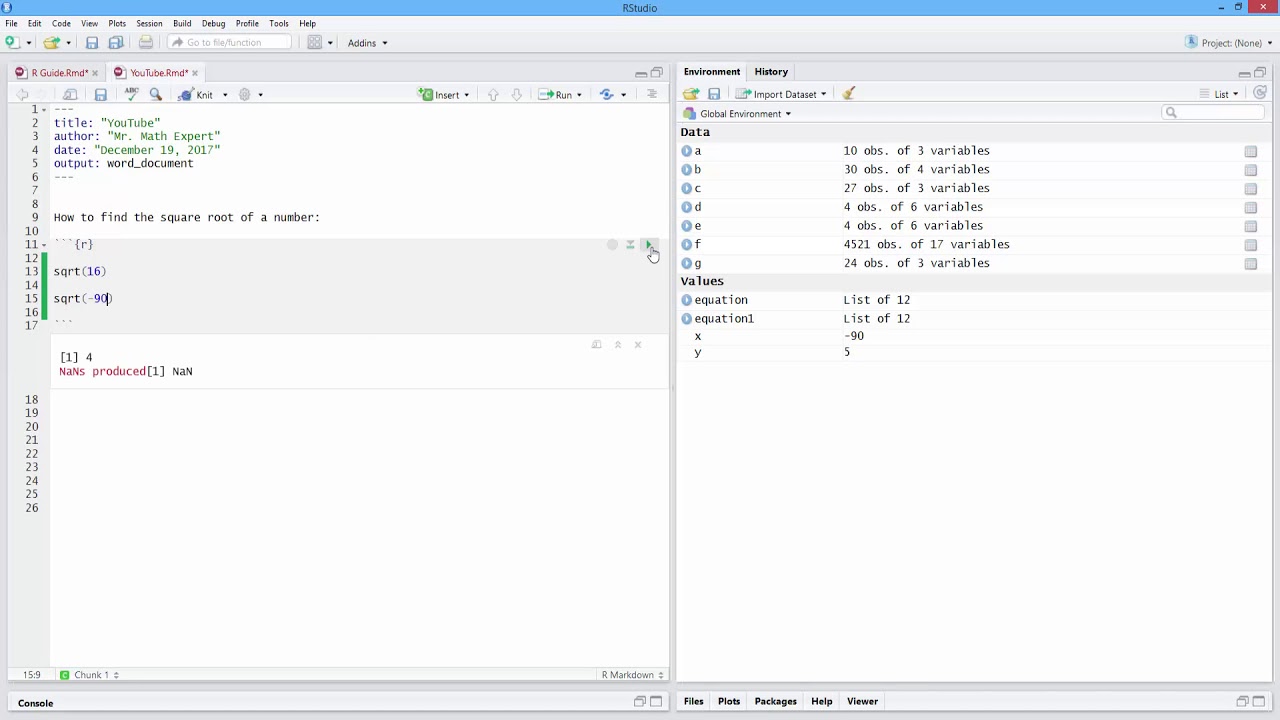
Vectorized Operations
In R, vectorized operations allow you to perform operations on entire vectors or matrices efficiently without using explicit loops. When applying the square root function sqrt()
to vectors or matrices:
- Create a vector or matrix of numeric values.
- Apply the
sqrt()
function directly to the vector or matrix. - R will compute the square root of each element individually and return a vector or matrix of the same dimensions with the results.
Example:
# Vectorized square root operation
numbers <- c(4, 9, 16, 25)
sqrt_numbers <- sqrt(numbers)
print(sqrt_numbers) # Output: [1] 2 3 4 5
This capability is particularly useful in data analysis and statistical computations where you often need to perform operations on large datasets efficiently.
Mathematical Functions Related to Square Root
In addition to the basic square root function sqrt()
, R provides several other mathematical functions that are related to square roots:
exp()
: Computes the exponential function, which is related to the square root through the identity \( e^{0.5 \cdot \ln(x)} \).log()
: Computes the natural logarithm, often used in transformations related to square roots.abs()
: Computes the absolute value, useful in scenarios involving complex numbers and their magnitudes.sqrt.default()
: A variant ofsqrt()
that handles missing values and non-numeric inputs differently.
These functions extend the utility of square root calculations to various mathematical and statistical applications in R.
Handling Missing Values
In R, handling missing values when calculating square roots or performing mathematical operations is crucial to ensure accurate results. Here are some techniques:
- Checking for NA values: Before computing square roots, use functions like
is.na()
to identify missing values in your dataset. - Filtering out missing values: Use
na.omit()
orcomplete.cases()
to exclude rows with missing values from your dataset. - Replacing missing values: Utilize
na.replace()
orifelse()
to substitute NA values with zeros or other appropriate replacements. - Handling NA results: If your calculations result in NA due to complex numbers or invalid inputs, consider using
na.rm = TRUE
in functions likesqrt()
to ignore NA values.
By applying these methods, you can effectively manage missing data when dealing with square roots and ensure robustness in your R programming projects.
Performance Considerations
When working with square roots in R, optimizing performance can enhance the efficiency of your code. Here are some strategies:
- Vectorization: Utilize vectorized operations whenever possible to compute square roots for entire vectors or matrices at once, rather than looping through individual elements.
- Benchmarking: Use benchmarking tools like
microbenchmark
to compare the execution time of different approaches and identify bottlenecks. - Data Structure Selection: Choose appropriate data structures such as arrays or data frames that are optimized for numerical computations.
- Pre-computation: Precompute square roots of constants or frequently used values to avoid redundant calculations during runtime.
- Package Selection: Consider using specialized packages like
Rcpp
for computationally intensive tasks to leverage C++ performance within R.
By implementing these techniques, you can significantly improve the speed and efficiency of square root calculations in your R scripts, particularly when dealing with large datasets or complex computations.

Visualizing Square Root Functions
Visualizing square root functions in R can provide insights into their behavior and relationships. Here are effective methods:
- Basic Plots: Use
plot()
to create simple plots of square root functions, showing how they relate to the original values. - Enhanced Visualizations: Utilize
ggplot2
for more advanced and customizable plots, adding features like trend lines and annotations. - Comparative Plots: Plot square root functions alongside original data or other transformations to visualize their effects on different datasets.
- Interactive Graphics: Create interactive plots using
plotly
orshiny
to explore square root functions dynamically with user inputs. - 3D Visualizations: For multidimensional data, use
rgl
orplot3D
to visualize square root transformations in three dimensions.
By employing these visualization techniques, you can gain a clearer understanding of square root functions and effectively communicate their outcomes in your R analyses.
Advanced Techniques and Tips
Exploring advanced techniques and tips can elevate your use of square root functions in R to the next level. Here’s a comprehensive approach:
- Custom Functions: Create custom functions using
function()
to encapsulate complex square root calculations with specific handling of edge cases. - Parallel Processing: Utilize
parallel
package for parallel computation of square roots across multiple cores or nodes, enhancing performance for large datasets. - Functional Programming: Apply functional programming concepts with
purrr
to efficiently apply square root operations to lists or data frames. - Error Handling: Implement robust error handling using
tryCatch()
to manage unexpected situations when computing square roots, ensuring graceful degradation. - Optimization Techniques: Use optimization algorithms like
optim()
to find optimal parameters in mathematical models involving square roots.
By mastering these advanced techniques, you can leverage the full potential of square root functions in R, making your code more efficient, scalable, and adaptable to diverse analytical challenges.
Resources for Further Learning
Exploring additional resources can deepen your understanding of square root functions in R. Here are valuable avenues for further learning:
- Official Documentation: Visit the official R documentation on square root functions for detailed syntax and usage examples.
- Online Courses: Enroll in platforms like Coursera or DataCamp for courses specifically covering mathematical functions in R.
- Books: Consult books such as "Advanced R" by Hadley Wickham for comprehensive insights into advanced R programming techniques.
- Community Forums: Engage with the R community on forums like Stack Overflow or RStudio Community to seek advice and share experiences.
- YouTube Tutorials: Watch tutorials from channels like "R Programming" or "Data Science Dojo" for practical demonstrations and tips.
By utilizing these resources, you can enhance your proficiency in using square root functions and broaden your knowledge of R programming.
Video này hướng dẫn chi tiết cách tìm căn bậc hai trong ngôn ngữ lập trình R. Hãy xem để nắm bắt thêm kiến thức về chủ đề này!
How to Find the Square Root in R - Hướng dẫn tìm căn bậc hai trong R
READ MORE:
Video này hướng dẫn chi tiết cách tính căn bậc hai của một số trong ngôn ngữ lập trình R và cách thực hiện điều này trong R Studio. Xem ngay để tìm hiểu thêm về chủ đề này!
Cách tính căn bậc hai của một số trong ngôn ngữ lập trình R | Hướng dẫn tính căn bậc hai trong R Studio