Topic square root in c: Discover how to effectively calculate the square root in C using the powerful sqrt() function from the math library. This comprehensive guide will walk you through the essential steps, best practices, and advanced techniques to ensure you master this fundamental aspect of C programming.
Table of Content
- Calculating Square Root in C
- Introduction to Square Root in C
- Including the Math Library in C
- Using the sqrt() Function
- Syntax of sqrt() Function
- Example Programs for Calculating Square Root
- Handling Negative Inputs with sqrt()
- Common Errors and Troubleshooting
- Optimizing Performance for Square Root Calculation
- Advanced Techniques for Square Root Calculation
- Applications of Square Root in C Programming
- Best Practices for Using sqrt() in C
- Conclusion and Summary
- YOUTUBE: Học cách tìm căn bậc hai bằng sqrt() sqrtf() sqrtl() trong lập trình C. Xem video hướng dẫn này để hiểu thêm về chủ đề căn bậc hai trong lập trình C.
Calculating Square Root in C
In C programming, calculating the square root of a number can be achieved using the sqrt()
function provided by the math library. Below, you'll find a detailed explanation of how to use this function along with example code.
Including the Math Library
To use the sqrt()
function, you need to include the math library in your program. This is done using the following preprocessor directive:
#include
Using the sqrt()
Function
The sqrt()
function takes a single argument of type double
and returns the square root of that argument. Here's the syntax:
double sqrt(double x);
Example Program
Below is an example program that demonstrates how to use the sqrt()
function to calculate the square root of a number:
#include
#include
int main() {
double number, result;
printf("Enter a number: ");
scanf("%lf", &number);
// Calculate the square root
result = sqrt(number);
printf("The square root of %.2f is %.2f\n", number, result);
return 0;
}
Important Notes
- If the argument passed to
sqrt()
is a negative number, the function will returnNaN
(Not a Number). - Always check if the input is valid to avoid unexpected results.
Using Mathjax for Mathematical Representation
If you want to represent the square root symbolically in HTML, you can use Mathjax. For example:
To represent the square root of x, you can use:
$$\sqrt{x}$$
This will display as:
$$\sqrt{x}$$
Conclusion
Calculating the square root in C is straightforward using the sqrt()
function from the math library. Remember to include the library and handle edge cases like negative inputs appropriately.

READ MORE:
Introduction to Square Root in C
Calculating the square root of a number is a fundamental operation in mathematics and programming. In C programming, computing the square root can be achieved using the sqrt()
function from the math library. This function returns the square root of a given number.
To use the sqrt()
function, you need to include the math library in your C program. This library provides various mathematical functions, including those for trigonometry, logarithms, and of course, square root calculation.
Understanding how to calculate square roots is essential for various applications, from basic mathematical computations to complex algorithms in fields like physics, engineering, and finance. Mastery of this function allows programmers to handle a wide range of numerical tasks efficiently.
This guide explores the usage of the sqrt()
function in C, covering its syntax, examples, handling of different input scenarios, common errors, optimization techniques, advanced calculation methods, practical applications, and best practices for implementation.
Including the Math Library in C
To use mathematical functions like square root calculation in C, you need to include the math library. Here’s how you can do it:
- Begin your C program with an include directive:
This line tells the compiler to include the math library, which contains various mathematical functions including#include
sqrt()
. - Compile your program with a command that links the math library:
Thegcc program.c -o program -lm
-lm
flag ensures that the math library is linked during compilation, enabling the use of functions likesqrt()
.
Once you've included the math library, you can start using mathematical functions in your C program to perform operations like square root calculation efficiently.
Using the sqrt() Function
The sqrt()
function in C is used to calculate the square root of a given number. Here’s how you can use it:
- Include the math library at the beginning of your C program:
This step is necessary to access the#include
sqrt()
function. - Call the
sqrt()
function in your program to compute the square root. For example:
This line computes the square root of 25 and stores the result in the variabledouble result = sqrt(25.0);
result
. - The
sqrt()
function takes a single argument of typedouble
and returns adouble
value which represents the square root.
Using sqrt()
allows you to perform square root calculations efficiently in C, making it a powerful tool for numerical computations and scientific programming.
Syntax of sqrt() Function
The syntax of the sqrt()
function in C is straightforward:
- Include the math library at the beginning of your C program:
This step is necessary to use the#include
sqrt()
function. - Call the
sqrt()
function where you want to calculate the square root:
Here,double sqrt(double x);
x
is the number whose square root you want to calculate. The function returns adouble
value which is the square root ofx
.
The sqrt()
function takes a single argument of type double
and returns a double
value representing the square root of the input.
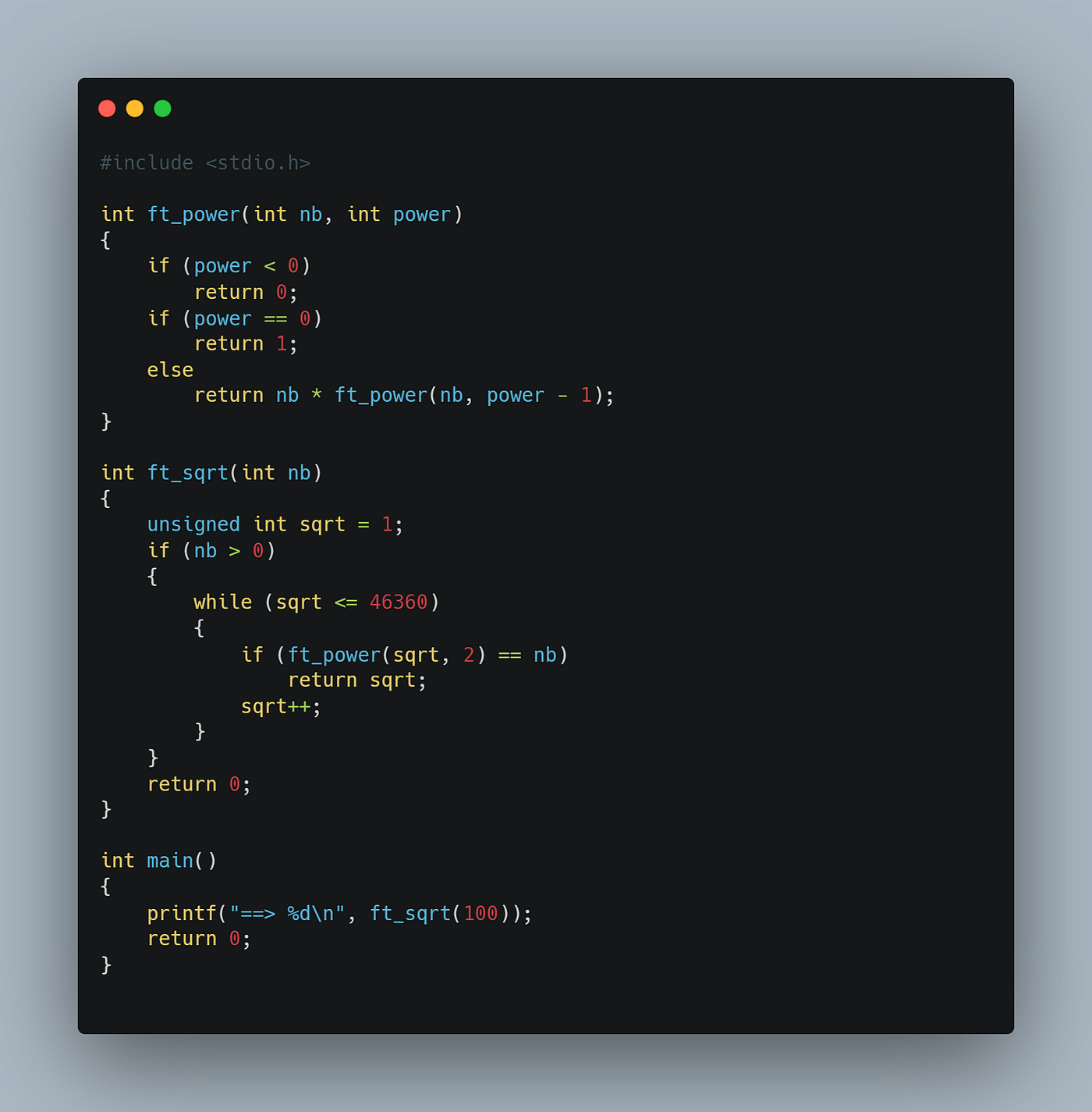
Example Programs for Calculating Square Root
Here are some example programs demonstrating how to calculate the square root using the sqrt()
function in C:
-
Basic program to compute the square root of a number:
#include
#include int main() { double number = 25.0; double result = sqrt(number); printf("Square root of %.2lf = %.2lf\\n", number, result); return 0; } This program calculates the square root of 25 and prints the result.
-
Program to calculate the square root of user-inputted numbers:
#include
#include int main() { double number, result; printf("Enter a number: "); scanf("%lf", &number); result = sqrt(number); printf("Square root of %.2lf = %.2lf\\n", number, result); return 0; } This program prompts the user to enter a number and calculates its square root.
These examples demonstrate how you can use the sqrt()
function in practical C programs to compute square roots efficiently.
Handling Negative Inputs with sqrt()
The sqrt()
function in C returns NaN (Not a Number) if it is passed a negative number. Here’s how you can handle negative inputs:
- Check if the input number is negative before calling
sqrt()
: - If the number is negative, handle the error condition appropriately in your program.
- You can print an error message, return an error code, or take other corrective actions depending on your application’s requirements.
- Example of handling negative input:
|
This program checks if the number is negative before computing its square root. If the number is negative, it prints an error message; otherwise, it calculates and displays the square root. |
By verifying the input before using sqrt()
, you can ensure your program handles potential errors gracefully when dealing with square root calculations in C.
Common Errors and Troubleshooting
When working with the sqrt()
function in C, you may encounter some common errors and issues:
- Undefined Reference to sqrt():
- This error occurs if you forget to include the math library at the beginning of your program using
#include
. - To fix this, ensure you include the math library to access the
sqrt()
function.
- This error occurs if you forget to include the math library at the beginning of your program using
- Handling Negative Numbers:
- If you pass a negative number to
sqrt()
, it returns NaN (Not a Number). - Ensure you validate input values before calling
sqrt()
to avoid such errors.
- If you pass a negative number to
- Incorrect Output:
- Double-check your input values and calculations to ensure you are getting the expected square root.
- Use printf statements to debug and verify intermediate results.
- Compiler Warnings:
- Pay attention to compiler warnings regarding type mismatches or implicit declarations.
- Resolve these warnings by ensuring correct data types and function declarations.
By understanding and addressing these common issues, you can troubleshoot and debug your C programs effectively when using the sqrt()
function for square root calculations.
Optimizing Performance for Square Root Calculation
Optimizing the performance of square root calculations in C can improve the efficiency of your programs. Here are some techniques:
- Minimize Square Root Calls:
- Avoid unnecessary calls to
sqrt()
by caching results or reusing computed values where possible. - Calculate square roots only when necessary to reduce computational overhead.
- Avoid unnecessary calls to
- Use Approximations:
- For applications where precise square roots aren’t crucial, consider using approximation techniques like Taylor series or iterative methods.
- These methods can be faster than the standard
sqrt()
function but may sacrifice accuracy.
- Precompute Values:
- If your program repeatedly computes square roots for a fixed set of inputs, precompute these values offline and store them for quick lookup during runtime.
- This approach reduces computation time at the expense of additional memory usage.
- Compiler Optimization:
- Enable compiler optimizations (e.g., using
-O2
or-O3
flags in GCC) to let the compiler optimize thesqrt()
function calls and improve overall performance. - Compiler optimizations can often inline functions or replace them with faster alternatives.
- Enable compiler optimizations (e.g., using
By implementing these optimization strategies, you can significantly enhance the efficiency of square root calculations in your C programs, ensuring they perform well even with large datasets or frequent mathematical computations.
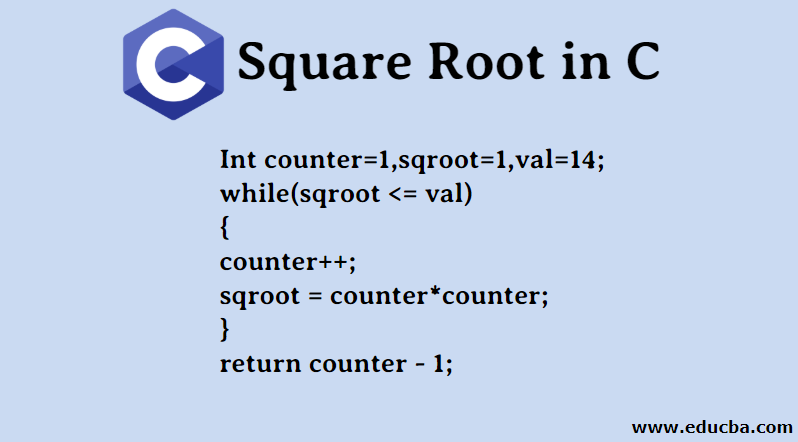
Advanced Techniques for Square Root Calculation
Advanced techniques for calculating square roots in C involve more sophisticated algorithms and methods beyond basic function calls. Here are some approaches:
- Newton-Raphson Method:
- This iterative method can be used to approximate square roots with improved accuracy and efficiency.
- It involves repeatedly refining an initial guess until the desired precision is achieved.
- Binary Search Method:
- In cases where the range of possible square roots is known, binary search can efficiently narrow down the correct square root.
- This method reduces the number of iterations compared to naive approaches.
- Integer Square Root:
- For calculating integer square roots (i.e., finding the largest integer whose square is less than or equal to a given number), specialized algorithms such as the Babylonian method can be utilized.
- These algorithms are optimized for integer calculations and can be faster than computing floating-point square roots.
- Approximation Algorithms:
- Various mathematical approximations and series expansions exist to compute square roots quickly, often sacrificing precision for speed.
- These algorithms are suitable for applications where approximate values suffice.
By exploring these advanced techniques, programmers can tailor square root calculations in C to specific performance and precision requirements, optimizing their applications for various computational tasks.
Applications of Square Root in C Programming
Square root calculations find numerous applications in C programming, ranging from mathematical computations to practical engineering and scientific simulations. Here are some key areas where the sqrt() function is commonly used:
- Numerical Analysis: In algorithms like Newton-Raphson for solving equations or in optimization techniques where root finding is essential.
- Physics and Engineering: Calculating magnitudes, velocities, accelerations, and other physical quantities involving square roots.
- Graphics: For transformations, scaling operations, and determining distances in 2D and 3D space.
- Statistics: Calculating standard deviations, which heavily rely on square roots.
- Signal Processing: Determining signal amplitudes and frequencies.
- Financial Calculations: In risk assessment and option pricing models.
- Machine Learning: Feature scaling and normalization processes.
These applications highlight the versatility and importance of the square root function in various domains of C programming, enhancing both numerical accuracy and computational efficiency.
Best Practices for Using sqrt() in C
When using the sqrt()
function in C for calculating square roots, it's essential to adhere to best practices to ensure accuracy and efficiency:
- Include the Math Library: Ensure to include the math library using
#include
at the beginning of your C program to access thesqrt()
function. - Handle Input Validation: Validate input values to
sqrt()
to prevent errors, especially for negative numbers which are not supported bysqrt()
. - Use Correct Data Types: Pass appropriate data types (like
double
for floating-point numbers) tosqrt()
to avoid truncation errors and ensure precise calculations. - Understand Precision: Recognize the limitations of floating-point arithmetic in C. For critical applications, consider alternative methods for calculating square roots with higher precision if necessary.
- Optimize Performance: Depending on your application, explore optimization techniques such as using precomputed lookup tables for square roots of commonly used values to enhance performance.
- Document and Comment: Clearly document the usage of
sqrt()
in your code, especially if algorithms or optimizations are employed, to aid understanding and maintenance. - Test Thoroughly: Test edge cases and boundary conditions to ensure that your implementation of
sqrt()
behaves correctly under all scenarios.
Conclusion and Summary
In conclusion, mastering the use of sqrt()
in C programming allows for efficient computation of square roots in various applications. Here’s a summary of key points:
- Function Integration: Integrate the math library with
#include
to accesssqrt()
. - Accuracy and Precision: Ensure correct data types and handle input validation to maintain accuracy.
- Performance Considerations: Optimize performance using suitable techniques like precomputed tables.
- Documentation and Testing: Document usage and thoroughly test implementations to guarantee reliability.
By following these practices, programmers can effectively leverage the sqrt()
function to enhance their C programming projects, achieving both accuracy and efficiency in square root calculations.
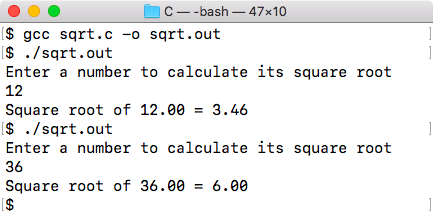
Học cách tìm căn bậc hai bằng sqrt() sqrtf() sqrtl() trong lập trình C. Xem video hướng dẫn này để hiểu thêm về chủ đề căn bậc hai trong lập trình C.
Find A Square Root Using sqrt() sqrtf() sqrtl() | Hướng dẫn lập trình C
READ MORE:
Học cách viết chương trình đơn giản trong C để tìm căn bậc hai của một số bất kỳ. Xem video này để hiểu thêm về cách sử dụng căn bậc hai trong lập trình C.
Chương trình đơn giản trong C để Tìm Căn Bậc Hai của Một Số Bất Kỳ