Topic square numbers java: Learn how to effectively square numbers in Java with this comprehensive guide. Whether you are using basic arithmetic, Java's Math library, or advanced techniques like BigDecimal for precision, this article covers all the methods to ensure accurate and efficient squaring operations. Start mastering squaring in Java today and elevate your coding skills!
Table of Content
- Methods to Square a Number in Java
- Conclusion
- Conclusion
- Introduction
- Basic Methods to Square a Number in Java
- Using Arithmetic Operator
- Using Math.pow() Method
- Using Math.multiplyExact() Method
- User-Defined Function for Squaring a Number
- Squaring Different Types of Numbers
- Handling Negative Numbers
- Handling Floating Point Numbers
- Example Programs
- Example 1: Using Math.pow()
- Example 2: Using User-Defined Function
- Example 3: Using multiplyExact()
- Example 4: Squaring a Number with User Input
- Best Practices and Tips
- YOUTUBE:
Methods to Square a Number in Java
- Using Multiplication Operator
This is the most straightforward method. You simply multiply the number by itself.
public class SquareNumber {
public static void main(String[] args) {
int number = 5;
int square = number * number;
System.out.println("The square of " + number + " is: " + square);
}
}
- Using Math.pow() Method
The Math.pow() method can also be used to square a number. This method is useful if you need to calculate higher powers as well.
public class SquareNumber {
public static void main(String[] args) {
double number = 5;
double square = Math.pow(number, 2);
System.out.println("The square of " + number + " is: " + square);
}
}
- Using multiplyExact() Method
The Math.multiplyExact() method is used to multiply two numbers and can be used to calculate the square of a number safely, avoiding overflow for integer types.
public class SquareNumber {
public static void main(String[] args) {
int number = 5;
int square = Math.multiplyExact(number, number);
System.out.println("The square of " + number + " is: " + square);
}
}
- Using a Custom Method
Creating a user-defined method to calculate the square can make the code cleaner and reusable.
public class SquareNumber {
public static void main(String[] args) {
int number = 5;
int square = square(number);
System.out.println("The square of " + number + " is: " + square);
}
public static int square(int num) {
return num * num;
}
}
- Using BigDecimal for Precision
When precision is critical, such as in financial calculations, using BigDecimal is preferred.
import java.math.BigDecimal;
public class SquareNumber {
public static void main(String[] args) {
BigDecimal number = new BigDecimal("5.5");
BigDecimal square = number.multiply(number);
System.out.println("The square of " + number + " is: " + square);
}
}
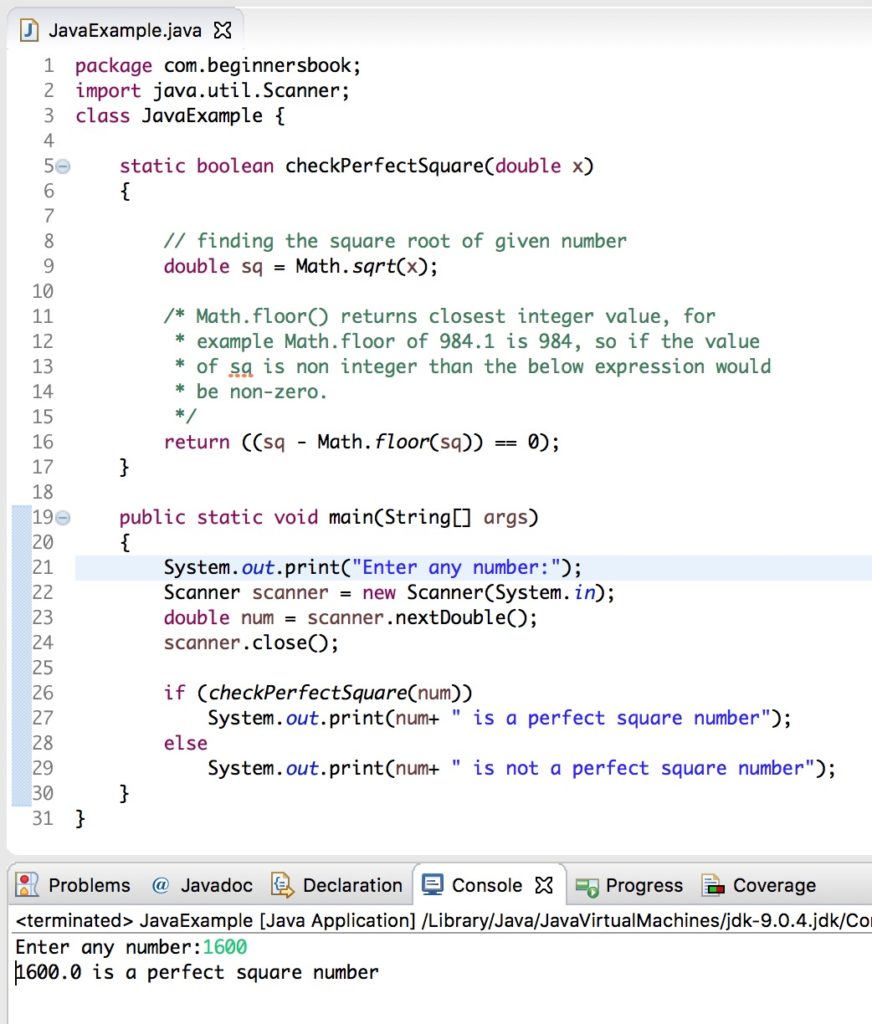
READ MORE:
Conclusion
There are various ways to square a number in Java, each suitable for different scenarios. The simplest method is using the multiplication operator, while Math.pow() offers more flexibility for calculating higher powers. For integer safety, Math.multiplyExact() is recommended, and for high precision, BigDecimal is the best choice. By practicing these methods, you can efficiently handle different requirements for squaring numbers in Java.
Conclusion
There are various ways to square a number in Java, each suitable for different scenarios. The simplest method is using the multiplication operator, while Math.pow() offers more flexibility for calculating higher powers. For integer safety, Math.multiplyExact() is recommended, and for high precision, BigDecimal is the best choice. By practicing these methods, you can efficiently handle different requirements for squaring numbers in Java.
Introduction
Squaring a number in Java is a fundamental operation that is often used in various mathematical and programming tasks. This operation involves multiplying a number by itself to produce its square. Java provides multiple ways to accomplish this, including using the `Math.pow()` function, simple multiplication, and user-defined methods. In this introduction, we will explore different methods to calculate the square of a number in Java, ensuring that you have a comprehensive understanding of each approach.
One of the simplest methods to square a number in Java is by using basic multiplication. This method is straightforward and efficient for most use cases:
int number = 5;
int square = number * number;
System.out.println("The square of " + number + " is: " + square);
Another common method is using the `Math.pow()` function, which can handle both integer and floating-point numbers. This function is part of the Java Standard Library and provides a versatile way to calculate powers:
double number = 3.5;
double square = Math.pow(number, 2);
System.out.println("The square of " + number + " is: " + square);
For more precise calculations, especially with large numbers or when dealing with high precision, the `BigDecimal` class can be used. This is particularly useful in financial and scientific applications where accuracy is crucial:
import java.math.BigDecimal;
BigDecimal number = new BigDecimal("5.5");
BigDecimal square = number.multiply(number);
System.out.println("The square of " + number + " is: " + square);
Additionally, Java allows for custom user-defined methods to encapsulate the logic of squaring a number, which can enhance code readability and reuse:
public class SquareNumber {
public static void main(String[] args) {
int number = 5;
int square = square(number);
System.out.println("The square of " + number + " is: " + square);
}
public static int square(int num) {
return num * num;
}
}
Understanding these different methods for squaring a number in Java will enable you to choose the most appropriate approach for your specific needs, ensuring both efficiency and accuracy in your programs.
Basic Methods to Square a Number in Java
Squaring a number in Java can be achieved through several methods, each offering unique benefits and applications. Below, we outline the most common techniques used to square numbers in Java.
-
Using Arithmetic Multiplication
This is the simplest and most direct method. It involves multiplying a number by itself.
int number = 5; int square = number * number; System.out.println("Square of " + number + " is: " + square);
-
Using the Math.pow() Method
The Math.pow() method from the Java Math library can also be used to calculate the square of a number. This method is more versatile as it can handle any power, not just squaring.
double number = 5; double square = Math.pow(number, 2); System.out.println("Square of " + number + " is: " + square);
-
Using the Math.multiplyExact() Method
The Math.multiplyExact() method ensures that the multiplication does not overflow, making it a safer option for squaring large numbers.
int number = 5; int square = Math.multiplyExact(number, number); System.out.println("Square of " + number + " is: " + square);
-
Using BigDecimal for Precision
For applications requiring high precision, such as financial calculations, the BigDecimal class can be used.
BigDecimal number = new BigDecimal("5"); BigDecimal square = number.multiply(number); System.out.println("Square of " + number + " is: " + square);
-
Loop-Based Multiplication
A loop-based approach, although less efficient, can be used for educational purposes or on platforms with limited capabilities.
int number = 5; int square = 0; for (int i = 0; i < number; i++) { square += number; } System.out.println("Square of " + number + " is: " + square);
-
Handling Negative Inputs
When squaring numbers, it's essential to handle negative inputs correctly. Squaring a negative number results in a positive output.
int number = -5; int square = number * number; System.out.println("Square of " + number + " is: " + square);

Using Arithmetic Operator
One of the simplest and most efficient ways to square a number in Java is by using the arithmetic multiplication operator. This method involves multiplying the number by itself. Here's a step-by-step guide on how to implement this approach:
- Declare an integer variable to store the number you want to square.
- Use the multiplication operator to multiply the number by itself.
- Store the result in another variable.
- Print the result to the console.
Here is a sample Java code demonstrating this method:
public class SquareNumber {
public static void main(String[] args) {
// The number to be squared
int number = 5;
// Calculate the square using the arithmetic operator
int square = number * number;
// Output the squared number
System.out.println("The square of " + number + " is: " + square);
}
}
This code initializes an integer variable number
with a value of 5, multiplies it by itself using the *
operator, and prints the result. This method is straightforward and executes quickly.
Using the arithmetic operator to square a number is particularly useful in scenarios where performance is critical, as this method avoids the overhead associated with method calls and type casting required by other methods like Math.pow()
.
Using Math.pow() Method
The Math.pow()
method is a versatile function provided by Java for various mathematical calculations, including squaring numbers. This method is part of the java.lang.Math
class and allows you to raise a number to the power of another number.
To use Math.pow()
for squaring a number, you need to pass two arguments to the method: the base number and the exponent. For squaring, the exponent will be 2. Below is a detailed step-by-step guide on how to use the Math.pow()
method to square a number in Java.
- Import the Math class:
Although the
Math
class is part of thejava.lang
package and doesn't require an explicit import, it's good practice to be aware of its location. - Declare and Initialize the Variable:
First, declare a variable to hold the number you want to square. You can initialize it with any value. For example:
double number = 5.0;
- Apply the
Math.pow()
Method:Use the
Math.pow()
method to calculate the square of the number. The method returns a double value, which can be cast to other types if necessary. Here is how you can do it:double squaredNumber = Math.pow(number, 2);
- Output the Result:
Finally, print the result to the console to verify the calculation:
System.out.println("The square of " + number + " is: " + squaredNumber);
Here is a complete example program:
public class SquareUsingMathPow {
public static void main(String[] args) {
// Declare and initialize the number
double number = 5.0;
// Calculate the square using Math.pow()
double squaredNumber = Math.pow(number, 2);
// Output the result
System.out.println("The square of " + number + " is: " + squaredNumber);
}
}
This example demonstrates the ease and efficiency of using the Math.pow()
method to square numbers in Java. The method is particularly useful for more complex calculations where raising a number to various powers is required.
Using Math.multiplyExact() Method
The Math.multiplyExact()
method in Java is a useful function for performing multiplication with built-in overflow detection. This method ensures that if the result of the multiplication exceeds the bounds of the data type, an ArithmeticException
is thrown, preventing incorrect calculations.
Syntax
public static int multiplyExact(int x, int y)
public static long multiplyExact(long x, long y)
public static long multiplyExact(long x, int y)
(introduced in JDK 9)
Parameters
x
: the first valuey
: the second value
Return Value
The method returns the product of x
and y
. If the result overflows, it throws an ArithmeticException
.
Example 1: Multiplication of Integers
public class MultiplyExactExample {
public static void main(String[] args) {
int a = 100;
int b = 20;
System.out.println(Math.multiplyExact(a, b)); // Output: 2000
}
}
Example 2: Multiplication of Longs
public class MultiplyExactExample {
public static void main(String[] args) {
long a = 1000L;
long b = 3000L;
System.out.println(Math.multiplyExact(a, b)); // Output: 3000000
}
}
Example 3: Handling Overflow
public class MultiplyExactExample {
public static void main(String[] args) {
try {
int a = 100000;
int b = 100000;
System.out.println(Math.multiplyExact(a, b));
} catch (ArithmeticException e) {
System.out.println("Overflow occurred: " + e.getMessage());
}
}
}
In this example, the result of the multiplication exceeds the maximum value an int
can hold, and thus an ArithmeticException
is thrown and caught in the catch block.
User-Defined Function for Squaring a Number
In Java, creating a user-defined function to square a number is a straightforward process. This allows for reusability and better code organization. Below is a detailed example demonstrating how to implement such a function.
- Define the function
- Call the function
Create a function called squareNumber
that takes an integer as a parameter and returns its square.
public static int squareNumber(int num) {
return num * num;
}
In the main
method, call the squareNumber
function with a user-provided input.
import java.util.Scanner;
public class SquareExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int number = scanner.nextInt();
int squared = squareNumber(number);
System.out.println("The square of " + number + " is: " + squared);
scanner.close();
}
}
This approach ensures that the logic for squaring a number is encapsulated within a single method, making the code modular and easy to maintain.
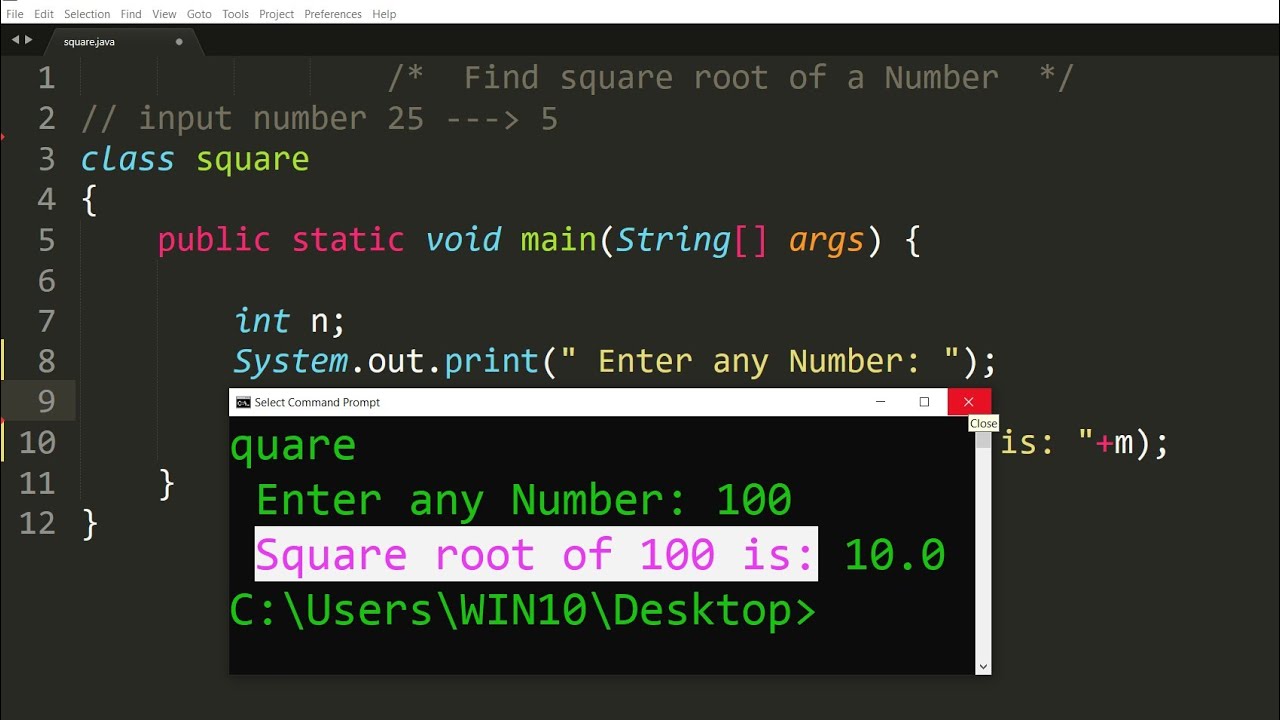
Squaring Different Types of Numbers
In Java, squaring a number can involve various data types including integers, floating-point numbers, and handling of special cases like negative numbers. Below are detailed methods for squaring different types of numbers:
1. Squaring Integers
To square an integer, you can simply multiply the integer by itself:
int number = 5;
int square = number * number;
System.out.println("The square of " + number + " is: " + square);
2. Squaring Floating-Point Numbers
For squaring floating-point numbers, you can use the same multiplication method or utilize the Math.pow()
method:
double number = 5.5;
double square = number * number;
System.out.println("The square of " + number + " is: " + square);
double squareWithPow = Math.pow(number, 2);
System.out.println("The square of " + number + " using Math.pow() is: " + squareWithPow);
3. Handling Negative Numbers
Squaring a negative number follows the same principles, resulting in a positive number:
int negativeNumber = -5;
int square = negativeNumber * negativeNumber;
System.out.println("The square of " + negativeNumber + " is: " + square);
4. Squaring Large Numbers
When dealing with large numbers, you can use the Math.multiplyExact()
method to avoid overflow issues:
long largeNumber = 100000L;
long square = Math.multiplyExact(largeNumber, largeNumber);
System.out.println("The square of " + largeNumber + " is: " + square);
5. Squaring Using User-Defined Functions
You can create a user-defined function to square numbers for better code reusability:
public class SquareCalculator {
public static int square(int number) {
return number * number;
}
public static double square(double number) {
return number * number;
}
public static void main(String[] args) {
int intNumber = 4;
double doubleNumber = 3.3;
System.out.println("Square of " + intNumber + " is: " + square(intNumber));
System.out.println("Square of " + doubleNumber + " is: " + square(doubleNumber));
}
}
Summary
- Integer squaring is done by simple multiplication.
- Floating-point squaring can be done using multiplication or
Math.pow()
. - Negative numbers yield positive squares.
- For large numbers,
Math.multiplyExact()
prevents overflow. - User-defined functions enhance code modularity and reusability.
Handling Negative Numbers
Squaring negative numbers in Java follows the same principles as squaring positive numbers. The result of squaring a negative number is always a positive number because a negative number multiplied by itself results in a positive product. Let's explore different methods to square negative numbers in Java:
- Using the Arithmetic Operator
*
- Using the
Math.pow()
Method - Using a User-Defined Function
Using the Arithmetic Operator *
This is the simplest method to square a number in Java. You can multiply the number by itself directly.
public class SquareNegative {
public static void main(String[] args) {
int number = -5;
int square = number * number;
System.out.println("Square of " + number + " is: " + square);
}
}
In this example, squaring -5 yields 25 because -5 * -5 = 25.
Using the Math.pow()
Method
The Math.pow()
method is a built-in Java function that can be used to calculate the power of a number.
public class SquareNegativeUsingPow {
public static void main(String[] args) {
double number = -4;
double square = Math.pow(number, 2);
System.out.println("Square of " + number + " is: " + square);
}
}
The Math.pow()
method takes two arguments: the base and the exponent. In this case, Math.pow(-4, 2)
returns 16.0.
Using a User-Defined Function
You can also create your own function to square a number. This approach allows for more flexibility and reusability.
public class UserDefinedSquare {
public static int square(int number) {
return number * number;
}
public static void main(String[] args) {
int number = -7;
int square = square(number);
System.out.println("Square of " + number + " is: " + square);
}
}
Here, the user-defined function square()
multiplies the input number by itself and returns the result. For an input of -7, the output is 49.
In summary, squaring a negative number in Java can be achieved using simple multiplication, the Math.pow()
method, or a user-defined function. Regardless of the method, the result will always be positive as the product of two negative numbers is positive.
Handling Floating Point Numbers
In Java, handling floating-point numbers requires careful consideration due to their inherent precision and rounding issues. Here are the key points and best practices for working with floating-point numbers:
1. Understanding Floating-Point Representation
Floating-point numbers are represented in two parts: the mantissa and the exponent. The Java primitive types for floating-point numbers are float
and double
. The double
type provides more precision than float
and is generally preferred for most calculations.
2. Arithmetic Operations
Java supports arithmetic operations such as addition, subtraction, multiplication, and division for floating-point numbers. However, it's important to be aware of potential rounding errors and loss of precision.
- Addition:
double sum = 3.14 + 2.71;
- Subtraction:
double difference = 3.14 - 2.71;
- Multiplication:
double product = 3.14 * 2.71;
- Division:
double quotient = 3.14 / 2.71;
3. Type Conversion and Casting
Conversions between floating-point types and other numeric types in Java can be done using casting. Explicit casting is required when converting from a larger data type to a smaller one to avoid data loss.
- Implicit casting:
float floatValue = 3.14F; double doubleValue = floatValue;
- Explicit casting:
double largeValue = 3.141592653589793; float smallFloat = (float) largeValue;
4. Using Math Functions and Constants
Java’s Math
class provides various mathematical functions and constants to perform complex operations with floating-point numbers.
- Square root:
double squareRoot = Math.sqrt(16.0);
- Exponentiation:
double power = Math.pow(2.0, 3.0);
- Trigonometric functions:
double sine = Math.sin(Math.PI / 2);
5. Handling Precision Issues
Due to finite precision, some decimal values cannot be represented exactly, leading to rounding errors. Here are some best practices:
- Use a small tolerance value for comparisons:
boolean isEqual = Math.abs(a - b) < 1e-9;
- Avoid using floating-point numbers in loops or conditional statements due to potential rounding errors.
- Consider using
BigDecimal
for monetary calculations or when high precision is required.
6. Example: Squaring a Floating-Point Number
Here is an example of squaring a floating-point number using the Math.pow()
method:
double number = 3.14;
double squared = Math.pow(number, 2);
System.out.println("Squared value: " + squared);
By following these guidelines, you can handle floating-point numbers effectively in Java, ensuring accurate and reliable calculations.
Example Programs
In this section, we will explore several example programs to demonstrate different ways to square numbers in Java. Each example showcases a unique approach to help you understand various methods of squaring numbers.
Example 1: Using Math.pow()
This example uses the Math.pow()
method to calculate the square of a number.
public class SquareUsingMathPow {
public static void main(String[] args) {
int number = 5;
double square = Math.pow(number, 2);
System.out.println("Square of " + number + " is: " + square);
}
}
In this program, we use Math.pow()
to calculate the square of the number 5
, and the result is printed to the console.
Example 2: Using a User-Defined Function
This example demonstrates how to create and use a user-defined function to square a number.
public class SquareUsingFunction {
public static int square(int num) {
return num * num;
}
public static void main(String[] args) {
int number = 7;
int result = square(number);
System.out.println("Square of " + number + " is: " + result);
}
}
Here, we define a function square()
that takes an integer as input and returns its square. This function is called in the main
method to square the number 7
.
Example 3: Using Math.multiplyExact()
This example uses the Math.multiplyExact()
method to ensure precise arithmetic operations without overflow.
public class SquareUsingMultiplyExact {
public static void main(String[] args) {
int number = 9;
int square = Math.multiplyExact(number, number);
System.out.println("Square of " + number + " is: " + square);
}
}
The Math.multiplyExact()
method is used to calculate the square of the number 9
, and the result is printed to the console.
Example 4: Squaring a Number with User Input
This example demonstrates how to read a number from user input and calculate its square.
import java.util.Scanner;
public class SquareWithUserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter a number: ");
int number = scanner.nextInt();
int square = number * number;
System.out.println("Square of " + number + " is: " + square);
}
}
In this program, a Scanner
object is used to read an integer input from the user. The square of the entered number is then calculated and printed.
These examples demonstrate various methods to square numbers in Java, providing a comprehensive understanding of different approaches. Whether you prefer using built-in methods or writing your own functions, these examples cover all basic scenarios.
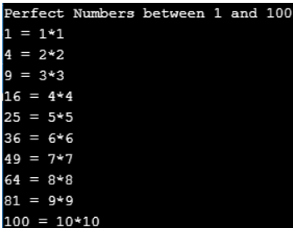
Example 1: Using Math.pow()
In this example, we demonstrate how to square a number in Java using the Math.pow()
method. This method is part of the java.lang.Math
class and allows us to raise a number to the power of another number.
Step-by-Step Guide
-
Import the
java.lang.Math
package:import java.lang.Math;
-
Create a class and a main method:
public class SquareExample { public static void main(String[] args) { // Your code here } }
-
Initialize the base number you want to square:
double base = 5.0;
-
Use the
Math.pow()
method to calculate the square:double result = Math.pow(base, 2);
-
Print the result:
System.out.println("The square of " + base + " is: " + result);
Complete Example
import java.lang.Math;
public class SquareExample {
public static void main(String[] args) {
double base = 5.0;
double result = Math.pow(base, 2);
System.out.println("The square of " + base + " is: " + result);
}
}
When you run this program, it will output:
The square of 5.0 is: 25.0
Additional Examples
You can also square different types of numbers such as floating-point numbers and negative numbers. Here are a few more examples:
Example with a Floating-Point Number
public class SquareFloat {
public static void main(String[] args) {
double base = 4.5;
double result = Math.pow(base, 2);
System.out.println("The square of " + base + " is: " + result);
}
}
Output:
The square of 4.5 is: 20.25
Example with a Negative Number
public class SquareNegative {
public static void main(String[] args) {
double base = -3.0;
double result = Math.pow(base, 2);
System.out.println("The square of " + base + " is: " + result);
}
}
Output:
The square of -3.0 is: 9.0
Conclusion
Using the Math.pow()
method in Java is a straightforward way to square a number. This method is versatile and can handle various types of numbers, including integers, floating-point numbers, and negative numbers.
Example 2: Using User-Defined Function
In Java, you can create user-defined functions to calculate the square of a number. This approach allows you to encapsulate the squaring logic in a reusable method. Here is a step-by-step guide to creating and using a user-defined function for squaring a number.
- Create a class to contain your methods.
- Define a static method that takes an integer as an argument and returns its square.
- In the main method, call the user-defined function and print the result.
Here is a sample code:
public class SquareCalculator {
// User-defined function to calculate the square
public static int calculateSquare(int number) {
return number * number;
}
public static void main(String[] args) {
int number = 7;
int square = calculateSquare(number);
System.out.println("The square of " + number + " is: " + square);
}
}
Let's break down the code:
- The
SquareCalculator
class contains the squaring logic. - The
calculateSquare
method is a user-defined method that calculates the square of a given number by multiplying the number by itself. - In the
main
method, we define a number and callcalculateSquare
to get its square. - The result is printed to the console using
System.out.println
.
This example demonstrates the simplicity and reusability of user-defined functions in Java.
Example 3: Using multiplyExact()
The Math.multiplyExact()
method in Java is a safe way to multiply two integers. It ensures that if the result overflows the data type, an ArithmeticException
is thrown. This is particularly useful when squaring a number, as it prevents overflow issues that could lead to incorrect results.
Below is an example program demonstrating how to square a number using the Math.multiplyExact()
method:
public class SquareUsingMultiplyExact {
public static void main(String[] args) {
int number = 5;
int square = squareNumber(number);
System.out.println("The square of " + number + " is: " + square);
}
public static int squareNumber(int num) {
return Math.multiplyExact(num, num);
}
}
Let's break down the code step-by-step:
-
Define the main class and method: The
SquareUsingMultiplyExact
class contains themain
method, which is the entry point of the program. -
Initialize the number: An integer variable
number
is initialized with the value you want to square, in this case, 5. -
Calculate the square: The
squareNumber
method is called withnumber
as an argument. This method usesMath.multiplyExact(num, num)
to calculate the square of the number safely. -
Print the result: The result is printed to the console with a descriptive message.
-
Define the squareNumber method: The
squareNumber
method takes an integernum
as a parameter and returns the square ofnum
using theMath.multiplyExact()
method.
Using Math.multiplyExact()
is particularly advantageous in applications where it is critical to handle integer overflows and ensure the correctness of mathematical operations.
Example 4: Squaring a Number with User Input
In this example, we will demonstrate how to take a number as input from the user and calculate its square using the Scanner
class in Java. The program will prompt the user to enter a number, read the input, and then display the squared result.
- Import Required Classes
First, we need to import the necessary classes:
import java.util.Scanner;
- Initialize the Scanner
Create an instance of the
Scanner
class to read input from the console:Scanner scanner = new Scanner(System.in);
- Prompt User for Input
Ask the user to enter a number:
System.out.print("Please enter a number: "); int number = scanner.nextInt();
- Calculate the Square
Compute the square of the input number:
int square = number * number;
- Display the Result
Output the result to the console:
System.out.println("The square of " + number + " is: " + square);
The complete Java program looks like this:
import java.util.Scanner;
public class SquareNumber {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter a number: ");
int number = scanner.nextInt();
int square = number * number;
System.out.println("The square of " + number + " is: " + square);
}
}
When you run this program, it will prompt you to enter a number, and then it will calculate and display the square of that number.
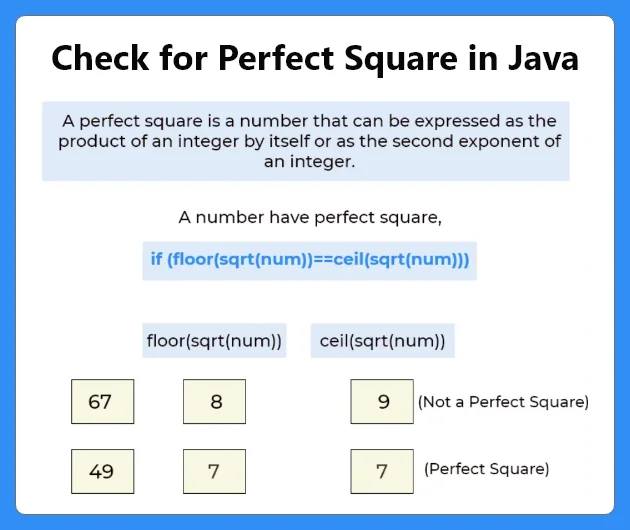
Best Practices and Tips
When squaring numbers in Java, following best practices and tips ensures efficiency, accuracy, and maintainability. Here are some key recommendations:
-
Use Appropriate Methods:
-
For basic squaring operations, using the multiplication operator
*
is the simplest and fastest method.int square = number * number;
-
For more complex cases, such as squaring floating-point numbers, consider using
Math.pow()
.double square = Math.pow(number, 2);
-
To handle large integer values and avoid overflow, use
Math.multiplyExact()
.int square = Math.multiplyExact(number, number);
-
-
Handle Negative Numbers:
Squaring a negative number results in a positive number. Ensure this is accounted for in your logic, particularly if negative inputs are unexpected or should be handled differently.
int number = -5; int square = number * number; System.out.println(square); // Outputs 25
-
Optimizing for Performance:
-
When dealing with powers of two, bit shifting can be a faster alternative.
int number = 4; int square = number << 1; // Equivalent to number * 2 System.out.println(square); // Outputs 8
-
Use
IntStream
for batch operations and to leverage parallel processing.IntStream.range(1, 6) .map(n -> n * n) .forEach(System.out::println); // Outputs: 1, 4, 9, 16, 25
-
-
Consider Precision:
For high precision calculations, especially with decimal values, use
BigDecimal
.BigDecimal bdValue = new BigDecimal("5.5"); BigDecimal square = bdValue.multiply(bdValue); System.out.println(square); // Outputs 30.25
-
Edge Cases and Error Handling:
Implement checks and handle cases such as zero, negative numbers, and very large values to prevent unexpected behavior or errors.
if (number < 0) { System.out.println("Please enter a non-negative number."); } else { int square = number * number; System.out.println(square); }
By adhering to these best practices, you can ensure your code for squaring numbers in Java is robust, efficient, and reliable.
Hướng dẫn Java - 15 - Lũy thừa và Căn bậc hai (Hàm toán học)
READ MORE:
Chương trình Java để tìm bình phương của số đã cho