Topic square root js: Understanding how to calculate the square root in JavaScript is crucial for developers working with numerical data. The Math.sqrt() function provides a straightforward way to find the square root of both positive integers and decimals, ensuring accurate and efficient calculations. Dive into our comprehensive guide to learn about the syntax, examples, and handling edge cases in JavaScript.
Table of Content
- JavaScript Square Root Calculation
- Introduction
- Using Math.sqrt() Method
- Handling Different Data Types
- Custom Implementation of Square Root Function
- Common Errors and Edge Cases
- Examples and Practical Applications
- Performance and Efficiency
- Conclusion
- YOUTUBE: Học cách tìm căn bậc hai trong Javascript mà không cần sử dụng Math.sqrt(). Video này sẽ hướng dẫn bạn từng bước để thực hiện điều đó một cách hiệu quả.
JavaScript Square Root Calculation
In JavaScript, calculating the square root of a number is straightforward using the built-in Math.sqrt()
method. This function is part of the Math object, which provides various mathematical functions and constants.
Usage of Math.sqrt()
The Math.sqrt()
method takes a single parameter, a number, and returns its square root. If a negative number or a non-numeric value is passed, the function returns NaN (Not-a-Number).
Syntax
Math.sqrt(x);
Where x
is the number for which you want to find the square root.
Examples
Square root of a positive number:
let val = 49; console.log(Math.sqrt(val)); // 7
Square root of a floating-point number:
let val = 5.14; console.log(Math.sqrt(val)); // 2.266...
Square root of a negative number:
let val = -9; console.log(Math.sqrt(val)); // NaN
Square root of a number passed as a string:
let val = '36'; console.log(Math.sqrt(val)); // 6
Square root of a non-numeric string:
let val = 'two'; console.log(Math.sqrt(val)); // NaN
Algorithm for Calculating Square Root without Math.sqrt()
You can also calculate the square root of a number without using the Math.sqrt()
method. Below is an example of a custom function that uses the iterative method:
function square_root(num) {
if (num < 0 || isNaN(num)) {
return NaN;
}
let square_root = num / 2;
let temp = 0;
while (square_root != temp) {
temp = square_root;
square_root = (num / square_root + square_root) / 2;
}
return square_root;
}
let positive_number = 121;
let negative_number = -6;
console.log("The square root of " + positive_number + " is: " + square_root(positive_number));
console.log("The square root of " + negative_number + " is: " + square_root(negative_number));
Benefits of Using Math.sqrt()
- Simple and easy to use
- Reliable and well-tested
- Efficient for performance
- No need for external libraries
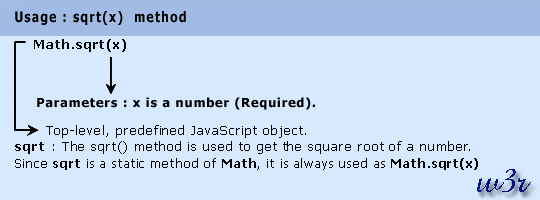
READ MORE:
Introduction
JavaScript offers various methods to calculate the square root of a number, providing developers with flexibility and precision. The most commonly used method is Math.sqrt()
, which computes the square root of a specified number. Additionally, there are alternative approaches like using Math.pow()
for more complex calculations or leveraging libraries such as decimal.js
for high precision. In this section, we'll explore these methods and their practical applications.
Math.sqrt()
method: This built-in JavaScript function takes a single numeric argument and returns its square root.Math.pow()
method: By raising a number to the power of 0.5, we can calculate its square root.- Using external libraries: For high precision, libraries like
decimal.js
provide robust solutions. - Custom algorithms: Implementing iterative methods to compute square roots without built-in functions offers more control over the process.
Understanding these methods allows developers to choose the most suitable approach for their specific use case, ensuring both accuracy and performance in their applications.
Using Math.sqrt() Method
The Math.sqrt()
method in JavaScript is a built-in function used to calculate the square root of a number. It is part of the Math object and is accessed using the syntax Math.sqrt(number)
. This method returns the square root of a positive number or decimal, and NaN
(Not a Number) for non-numeric or negative values.
Here are the steps and examples to effectively use Math.sqrt()
:
-
Square Root of a Positive Integer:
let result = Math.sqrt(16); // result is 4
-
Square Root of a Decimal Number:
let result = Math.sqrt(25.64); // result is approximately 5.064
-
Handling Negative Numbers:
let result = Math.sqrt(-9); // result is NaN
-
Handling Non-Numeric Strings:
let result = Math.sqrt('hello'); // result is NaN
-
Handling Numeric Strings:
let result = Math.sqrt('36'); // result is 6
-
Square Root of Infinity Values:
let result = Math.sqrt(Infinity); // result is Infinity
let result = Math.sqrt(-Infinity); // result is NaN
Examples of practical applications:
- Calculate the square root of user input:
const number = prompt('Enter the number:');
const result = Math.sqrt(number);
console.log(`The square root of ${number} is ${result}`); - Square root of an array with a single element:
let numArray = [49];
console.log(Math.sqrt(numArray)); // 7 - Square root of an empty string or null:
console.log(Math.sqrt('')); // 0
console.log(Math.sqrt(null)); // 0
The Math.sqrt()
method is a versatile and essential tool for mathematical calculations in JavaScript, ensuring that you can handle a variety of input types effectively.
Handling Different Data Types
When working with the Math.sqrt()
method in JavaScript, it is important to handle different data types appropriately to ensure accurate results and avoid potential errors. Below, we will explore how to manage various data types such as numbers, strings, arrays, and objects.
1. Numbers
The Math.sqrt()
method is designed to handle numerical values. When a number is passed to the method, it returns the square root of that number. For example:
let result = Math.sqrt(16); // result is 4
2. Strings
If a string containing a numerical value is passed to Math.sqrt()
, JavaScript will attempt to convert the string to a number before calculating the square root. However, if the string cannot be converted to a number, the method will return NaN
(Not-a-Number). For example:
let result1 = Math.sqrt("25"); // result1 is 5
let result2 = Math.sqrt("abc"); // result2 is NaN
3. Arrays
When an array is passed to the Math.sqrt()
method, JavaScript first tries to convert the array to a single numerical value. If the array has one element that is a number or a string representing a number, it will return the square root of that element. If the array is empty or has more than one element, the method returns NaN
. For example:
let result1 = Math.sqrt([9]); // result1 is 3
let result2 = Math.sqrt([]); // result2 is NaN
let result3 = Math.sqrt([1, 2]); // result3 is NaN
4. Objects
Passing an object to Math.sqrt()
will result in NaN
because objects cannot be directly converted to a numerical value. For example:
let result = Math.sqrt({value: 4}); // result is NaN
5. Special Cases
It is also important to handle special cases such as negative numbers and non-numeric values:
- For negative numbers,
Math.sqrt()
returnsNaN
because the square root of a negative number is not a real number. - For
null
,Math.sqrt(null)
returns 0 becausenull
is converted to 0. - For
undefined
,Math.sqrt(undefined)
returnsNaN
.
let result1 = Math.sqrt(-1); // result1 is NaN
let result2 = Math.sqrt(null); // result2 is 0
let result3 = Math.sqrt(undefined); // result3 is NaN
By understanding how Math.sqrt()
handles different data types, you can ensure that your code accounts for various input scenarios, leading to more robust and error-free applications.
Custom Implementation of Square Root Function
In JavaScript, you can create a custom implementation of the square root function using methods like the Newton-Raphson method. This approach is useful when you need more control over the computation process or want to handle specific edge cases. Here is a step-by-step guide to creating a custom square root function:
Newton-Raphson Method
The Newton-Raphson method is an iterative technique for finding successively better approximations to the roots (or zeroes) of a real-valued function.
- Start with an initial guess for the square root.
- Iterate using the formula:
guess = (guess + value / guess) / 2
. - Continue the iterations until the guess stabilizes.
Example Implementation
Below is an example of how you can implement this method in JavaScript:
function newtonRaphsonSquareRoot(value) {
if (value < 0 || isNaN(value)) {
return NaN;
}
let guess = value / 2;
let temp;
do {
temp = guess;
guess = (guess + value / guess) / 2;
} while (guess !== temp);
return guess;
}
const number = 16;
const squareRoot = newtonRaphsonSquareRoot(number);
console.log(`The square root of ${number} using Newton-Raphson is ${squareRoot.toFixed(2)}`);
Step-by-Step Explanation
- Initialize: Begin with an initial guess, typically half of the input value.
- Iterate: Apply the Newton-Raphson formula repeatedly until the guess no longer changes significantly.
- Return: Once the guess stabilizes, return it as the square root.
Alternative Method
Another approach is to use a simple iterative method:
function customSquareRoot(num) {
if (num < 0 || isNaN(num)) {
return NaN;
}
let guess = num / 2;
let temp = 0;
while (guess !== temp) {
temp = guess;
guess = (num / guess + guess) / 2;
}
return guess;
}
console.log(customSquareRoot(121)); // Output: 11
console.log(customSquareRoot(-6)); // Output: NaN
This approach checks if the input is valid (non-negative and numeric), initializes the guess, and iterates until the guess value stabilizes, similar to the Newton-Raphson method.
Using Math.pow()
Method
For an alternative built-in solution, you can use the Math.pow()
function:
const number = 16;
const squareRoot = Math.pow(number, 0.5);
console.log(`The square root of ${number} using Math.pow() is ${squareRoot}`);
The Math.pow()
function raises a number to the power of 0.5 to find its square root.
These custom implementations provide more control and flexibility for specific use cases, while the built-in Math.sqrt()
and Math.pow()
functions offer simplicity and ease of use.

Common Errors and Edge Cases
When using the Math.sqrt()
method in JavaScript, it's important to handle common errors and edge cases to ensure robust and error-free code. Here are some of the most common scenarios to consider:
1. Handling Negative Numbers
The Math.sqrt()
method returns NaN
(Not-a-Number) for negative inputs, as the square root of a negative number is not a real number. You should check for negative inputs before calling the method:
function safeSqrt(x) {
if (x < 0) {
return NaN;
}
return Math.sqrt(x);
}
2. Zero and Negative Zero
Both 0
and -0
are valid inputs for Math.sqrt()
and will return 0
. However, distinguishing between these values might be necessary for certain applications:
Math.sqrt(0); // 0
Math.sqrt(-0); // -0
3. Non-Numeric Inputs
If the input to Math.sqrt()
is not a number, the method will return NaN
. It's good practice to validate inputs:
function safeSqrt(x) {
if (typeof x !== 'number' || isNaN(x)) {
return NaN;
}
return Math.sqrt(x);
}
4. Large Numbers
When dealing with very large numbers, the precision of the square root calculation can become an issue. JavaScript uses double-precision floating-point format, which can cause inaccuracies:
let largeNumber = 1e308;
console.log(Math.sqrt(largeNumber)); // Outputs a very large number but with possible precision loss
5. Infinity
The Math.sqrt()
method returns Infinity
when the input is Infinity
:
Math.sqrt(Infinity); // Infinity
6. Non-integer Inputs
The Math.sqrt()
method works with floating-point numbers as well. Ensure that you handle fractional values correctly:
Math.sqrt(2); // 1.4142135623730951
Math.sqrt(2.25); // 1.5
7. Handling Edge Cases in Testing
When writing tests for functions that use Math.sqrt()
, consider the following edge cases:
- Zero and Negative Zero
- Negative numbers
- Non-numeric values (e.g.,
null
,undefined
,NaN
) - Very large numbers and
Infinity
- Very small numbers (close to zero but negative)
By handling these common errors and edge cases, you can ensure that your use of the Math.sqrt()
method is reliable and robust in various scenarios.
Examples and Practical Applications
The concept of square roots is widely used in various real-life scenarios and practical applications. Here are some notable examples:
1. Finance
In finance, square roots are used to calculate stock market volatility. This metric helps investors understand the risk associated with a particular investment. By taking the square root of the variance of stock returns, investors can determine the standard deviation, which measures the extent of variation in the stock prices.
2. Engineering and Architecture
Square roots are essential in engineering, especially when determining the natural frequency of structures like bridges and buildings. The natural frequency helps predict how a structure will respond to various loads, such as wind or traffic, ensuring stability and safety.
3. Science
In science, square roots are used in numerous calculations, such as determining the velocity of a moving object, the intensity of sound waves, or the amount of radiation absorbed by a material. These calculations are crucial for understanding physical phenomena and developing new technologies.
4. Statistics
Statisticians use square roots to calculate standard deviation, which is the square root of the variance. This measure indicates how much data points deviate from the mean, providing insights into data distribution and variability.
5. Geometry
In geometry, square roots are used to compute the area and perimeter of shapes, solve problems involving right triangles, and apply the Pythagorean theorem. For example, calculating the hypotenuse of a right triangle requires finding the square root of the sum of the squares of the other two sides.
6. Computer Science
Square roots play a vital role in computer science, particularly in algorithms for encryption, image processing, and game physics. For instance, encryption algorithms use modular arithmetic and square roots to generate secure keys for data transmission.
7. Cryptography
Cryptographic techniques often employ square roots in digital signatures, key exchange systems, and secure communication channels. These applications ensure data authenticity and security in digital transactions.
8. Navigation
Square roots are used in navigation to compute distances between points on a map or globe. Pilots and sailors use these calculations to determine the shortest route and direction between two locations.
9. Electrical Engineering
In electrical engineering, square roots are used to calculate power, voltage, and current in circuits. These calculations are essential for designing and optimizing electrical systems and devices.
10. Photography
The aperture of a camera lens, which controls the amount of light entering the camera, is expressed as an f-number. The area of the aperture is proportional to the square of the f-number, influencing exposure and depth of field in photography.
11. Computer Graphics
In computer graphics, square roots are used to calculate distances between points and the length of vectors. These calculations are fundamental in rendering 2D and 3D graphics accurately.
12. Telecommunication
Square roots are used in telecommunication to model signal strength, which decreases with the square of the distance from the transmitter. This principle, known as the inverse square law, is crucial for designing effective communication networks.
These examples illustrate the wide-ranging applications of square roots in various fields, highlighting their importance in solving real-world problems and advancing technology.
Performance and Efficiency
The performance and efficiency of square root calculations in JavaScript are critical in applications requiring numerous or real-time computations. The Math.sqrt()
method is optimized for fast and accurate results, making it suitable for most use cases. Here are several key points to consider:
-
Native Performance: The
Math.sqrt()
function is implemented in the JavaScript engine's core, providing near-native performance. This makes it significantly faster than custom JavaScript implementations for calculating square roots. -
Algorithm Efficiency: The underlying algorithm for
Math.sqrt()
is highly optimized. It typically uses efficient techniques like the iterative Babylonian method (also known as Heron's method), ensuring a good balance between speed and precision. -
Browser Optimization: Different browsers may have slight variations in their JavaScript engines (e.g., V8 for Chrome, SpiderMonkey for Firefox). However, all modern browsers offer high efficiency for the
Math.sqrt()
function, thanks to ongoing optimizations. -
Handling Large Data Sets: When dealing with large arrays or data sets, using
Math.sqrt()
in combination with array methods (e.g.,map()
) ensures that the computations remain efficient and performant. For example:const numbers = [1, 4, 9, 16, 25]; const roots = numbers.map(Math.sqrt); console.log(roots); // Output: [1, 2, 3, 4, 5]
-
Custom Implementations: While custom implementations of the square root function can be educational or necessary for specific cases, they generally do not match the performance of
Math.sqrt()
due to the lack of low-level optimizations. Here's an example of a custom square root function using the Newton-Raphson method:function customSqrt(value, epsilon = 1e-10) { if (value < 0) throw new Error("Negative value"); let guess = value; while (Math.abs(guess * guess - value) > epsilon) { guess = (guess + value / guess) / 2; } return guess; } console.log(customSqrt(25)); // Output: 5
-
Precision Considerations: The precision of
Math.sqrt()
is typically sufficient for most applications. However, developers should be aware of potential floating-point precision issues in JavaScript when dealing with extremely large or small numbers. -
Benchmarking and Profiling: For performance-critical applications, it's essential to benchmark and profile your code. JavaScript performance tools available in modern browsers can help identify bottlenecks and optimize the use of
Math.sqrt()
within larger computational tasks.
In conclusion, Math.sqrt()
provides an efficient and performant way to compute square roots in JavaScript. Leveraging the built-in method ensures that your applications run smoothly, even when handling large-scale computations.
Conclusion
In conclusion, the Math.sqrt()
method in JavaScript is a simple, efficient, and reliable way to calculate the square root of a number. It handles a wide range of inputs, including positive numbers, negative numbers, and non-numeric values, returning the appropriate results or NaN
when necessary.
- Simplicity: The
Math.sqrt()
method is straightforward to use, making it accessible for developers at any skill level. You simply pass a number as an argument, and it returns the square root. - Reliability: As a built-in function,
Math.sqrt()
is thoroughly tested and provides accurate results within the limits of JavaScript's numerical precision. - Efficiency: This method is optimized for performance, making it suitable for applications that require numerous square root calculations, such as real-time data processing or scientific computations.
- No External Libraries Needed: Since it is a native JavaScript function, there is no need to include external libraries, reducing complexity and dependency management in your projects.
While Math.sqrt()
is generally the best choice for most applications, understanding alternative methods, like custom iterative approaches, can be beneficial for educational purposes or specific use cases where precision and custom behavior are needed.
Overall, the Math.sqrt()
method is an essential tool in JavaScript for any task that involves square root calculations, offering a balance of simplicity, reliability, and efficiency.

Học cách tìm căn bậc hai trong Javascript mà không cần sử dụng Math.sqrt(). Video này sẽ hướng dẫn bạn từng bước để thực hiện điều đó một cách hiệu quả.
Tìm căn bậc hai trong Javascript mà không dùng Math.sqrt()
READ MORE:
Học cách tính căn bậc hai của một số trong JavaScript. Video này sẽ hướng dẫn bạn cách thực hiện với các ví dụ cụ thể.
#3 Căn bậc hai của một số | Hướng dẫn JavaScript